- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
ten Best Real money Casinos on the internet for Us People in the 2025
4 February 2025 20x Uncategorized
Articles
Whenever to experience in the greatest online casinos the real deal currency, some have may be more significant than the others. Such as, payment rates and you will payment tips you will count more games possibilities. To be sure you are making a well-told decision, find out about the way we rate. Choose signed up casinos on the internet one to follow strict laws and regulations thereby applying state-of-the-art defense protocols to safeguard your own personal and you may financial advice.
Pennsylvania Betting Control panel
Choosing the better internet casino entails an extensive evaluation of a lot https://uk.noiseshape.com/song-bac-truc-tuyen-thanh-toan-nhanh-nhat-tai-hoa-ky-vao-nam-2025 important aspects to ensure a safe and you can satisfying gambling feel. Researching the brand new local casino’s character because of the learning analysis from trusted source and checking user views to your forums is an excellent first step. This helps you gain insight into the fresh feel away from other players and you may pick any possible points. No deposit bonuses in addition to enjoy prevalent prominence among marketing and advertising procedures.
Which are the best real money local casino sites?
Including, for individuals who bring 50 100 percent free spins and employ him or her for the a finest position game, you have made 50 totally free photos so you can house a commission instead of dipping in the real-currency gambling enterprise equilibrium. Gambling enterprises add to the enjoyable through providing position players 100 percent free spins, nice incentives, and other advantages. If you’d like to win large, progressive harbors and sexy-drop jackpots are some of the greatest online slots games you could play for real money in america. Bettors features other choices in terms of what a common games is. The brand new web based casinos live gives gamers the ability to enjoy any type of imaginable form of gaming. Should your favourite gambling establishment video game is actually slot machines, you’ll should see a slots gambling establishment.
A varied directory of high-high quality online game away from reputable application team is another crucial basis. Come across casinos offering numerous games, along with slots, dining table online game, and you will alive agent possibilities, to ensure you may have loads of choices and you will enjoyment. The fresh judge structure to possess United states of america gambling on line is within a stable condition away from flux.
The new excitement out of seeing the ball home on your chosen number otherwise colour try unrivaled. During the the casino website you’ll find different kinds of videos casino poker as well as jackpot casino poker, joker web based poker while some. These incentives give you right back a share of one’s losses more a certain time period. Cashback incentives will likely be a powerful way to recoup the your own losings and keep maintaining your to play. Washington passed laws to let online gambling inside the 2021, but it is primarily worried about sportsbooks. Delaware has legalized online gambling, but it’s seemingly small in the measure.
John’s love of creating gambling enterprise guides comes from their local casino experience along with his passion for permitting fellow punters. Their articles are over reviews; he could be narratives you to publication one another novices and you will seasoned participants because of the newest labyrinth of online casinos. Real time specialist playing the most immersive points readily available for the online a real income local casino web sites.
What are the better real cash online casinos inside the 2025?
The new Federal State Gaming Helpline now offers twenty four/7 label, text message, and you can talk characteristics, hooking up individuals with local information and organizations. Ignition Gambling enterprise, Bistro Local casino, and DuckyLuck Gambling enterprise provides obtained prizes to have Casino Driver of one’s 12 months, exemplifying its world identification and trustworthiness. Fans out of Roulette have the choice out of indulging in the fresh Eu and you will Western models. Per now offers a different number of regulations and you will game play enjoy, catering to several choices.
All of us from professionals features assessed and you will ranked the major United states internet casino websites you to strictly follow state and federal laws and regulations. Titles including Super Roulette, Front side Bet City, Local casino Hold’em, and In love Time rank because the some of the best alive agent video game in the industry. Baccarat as well as spends a blend of point totals, cards, and very easy wagers.
- Constantly read the small print understand the new wagering criteria and eligible games, making certain you could make the most of the added bonus.
- When you’re confirmation process is actually a fundamental defense size, they could both getting invasive.
- It also have an advisable loyalty system that have quick distributions in the the greater sections.
- In the event you take pleasure in inspired ports, game for example Mystical Wolf and you can Fantastic Buffalo give an enthusiastic immersive and you can humorous sense.
- You’ll find information about all licensees on the regulatory authorities’ certified websites.
- Here are our very own tips for to experience wise to help you provides enjoyable and increase your chances of successful big.
These systems offer a selection of distinctions, that have classics including Jacks otherwise Greatest demonstrating for example preferred. On the web real money gambling establishment internet sites is court in the six You.S. states, but four says stick out. Ney Jersey, Michigan, Pennsylvania, and you may Western Virginia established powerful, full-size locations.
- That have generous incentives and you will offers, it’s a substantial selection for players looking for a fun and you will fulfilling experience.
- Gamble casino black-jack in the Insane Gambling enterprise and choose out of a variety from alternatives in addition to four given, multi-give, and you will single deck black-jack.
- In this old-fashioned table games, you essentially wager on whether the user or even the banker have a tendency to score a rating closer to 9.
- They come is actually extremely claims, with some celebrated exceptions (constantly ID, MI, MT, NV, WA, with regards to the specific brand).
These types of limits assist participants manage what kind of cash transmitted otherwise invested in bets to the a daily, weekly, month-to-month, otherwise annual basis. Because of the form these constraints, participants can also be create their gambling things more effectively and get away from overspending. This type of bonuses typically matches a portion of the very first deposit, providing additional fund to play which have. Such, Las Atlantis Local casino also offers a good $2,five-hundred deposit matches and you may dos,five-hundred Reward Credit just after wagering $twenty-five within the basic seven days. Gambling enterprise gambling on the web will be challenging, however, this guide simplifies it.
Whether or not your’re also chasing huge jackpots or watching informal revolves, Slots LV suits all kinds of slot fans. A real income casinos not merely offer the thrill of potential huge earnings and also render a interesting experience with the additional limits. Progressive jackpots, including, can be drop at any given time, incorporating a supplementary level from adventure for the playing class. Information games statistics, such winnings prices and you can payment speeds, helps you make advised choices, enhancing your chances of effective. Starmania because of the NextGen Playing integrates visually excellent image with an enthusiastic RTP from 97.87%, making it a popular among players seeking to each other appearance and you will high profits. White Bunny Megaways away from Big-time Gaming now offers a great 97.7% RTP and you may an intensive 248,832 ways to earn, making certain a thrilling betting knowledge of nice commission possible.
Plenty of players who are looking poker, black colored jack, otherwise roulette choose to gamble in the an internet local casino who’s an alive agent feature. Now, a great deal of playing casinos try on the market which may be reached on line. A knowledgeable real cash on-line casino depends on info such as your financing method and and that online game you want to enjoy. For individuals who’lso are a good baccarat user, you’ll need to work on finding the optimum baccarat gambling enterprise on the internet. Poker players concurrently will want to look to have online casinos having great casino poker to play possibilities.
Invited, fellow excitement-seekers, on the pleasant market of web based casinos! Today, in just several presses, you could drench your self inside an exhilarating arena of betting and you will gaming from your house. When queries develop or issues can be found, dedicated assistance groups are available twenty-four hours a day that will help you.
If you claim a free of charge spins give with no deposit necessary, you’ll features to 20 extra revolves to play to your specific position video game for example Barcrest’s Rainbow Wealth. If a gambling establishment will give you 100 percent free revolves as an element of an excellent put bonus, the amount of spins is much big. An educated real cash casinos on the internet inside 2025 is actually Ignition Casino, Eatery Casino, and you will Bovada Gambling enterprise, noted for their nice bonuses, game variety, and you will best-level customer care.
Mungkin Anda tertarik membaca artikel berikut ini.
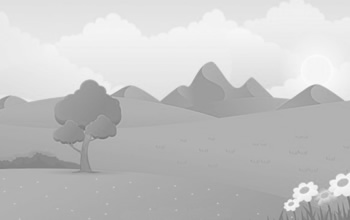
Non-British Gambling enterprises, Gambling enterprises Without Uk License 2024
Articles Freshbet sportsbook – ✅ 2: Find a suitable Banking Choice Examining the Advantages and disadvantages from Low Gamstop Gambling enterprises Only United kingdom Club Low Gamstop Casinos Book Sort of Internet casino Websites You can even fool around with Skrill otherwise Neteller for instantaneous places and you may small distributions. Blackja... selengkapnya
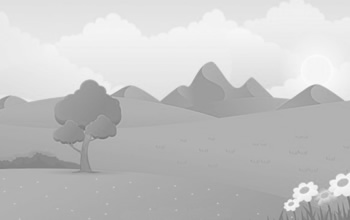
Мелбет промокод 2025 VR12: скидка до 15 000 руб.
Content Подпишись на закрытые промокоды а еще акции 💸 Перекусывать единица дли вас промокоды Мелбет (Melbet)? Дело через Melbet! Мастерите ставки на подобии «экспресс» вдобавок получите и распишитесь 10% ск... selengkapnya
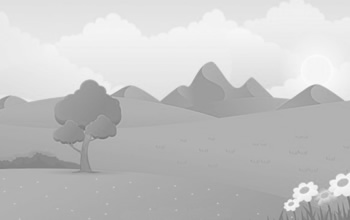
Finest United states Real money No deposit Local casino Bonuses 2025
Posts What to Look out for in the new No deposit Bonus Web based casinos United states of america No deposit versus. Fits Deposit Bonuses No-deposit bonuses usually are to possess position game, however they can often be applied to dining table video game such as blackjack, roulette, otherwise web based poker. Once making use... selengkapnya