- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Share On-line casino, Promo code for top level Added bonus 2025
5 February 2025 20x Uncategorized
Blogs
Check always local laws and regulations, because the state-particular regulations may differ, and in specific components, usage of the working platform might possibly be limited. In the claims where Risk.us is legal, players can enjoy a secure and you can entertaining on the web playing sense. This site stake.united states provides a person-friendly interface, that is just like the Stake site while offering a sort of web sites ports and you can dining table video game away from greatest developers. There are even certain extra also offers and you may promotions, which can be beautifully satisfying and you will unique for that Risk website extension.
🕹 Well-known Online casino games
This allows participants to verify the newest fairness of every move, spin, or draw to ensure that the results have not been controlled. However, i never ever seem to earn in almost any of your own online game, the degree of minutes i’ve destroyed inside the black-jack https://landspot.pl/2025/02/01/nhan-phan-thuong-duoc-moi-leovegas-cua-ho/ try disgusting even playing with the brand new black-jack method. Simply prefer a new login name, strong code, and supply your own email, identity, date from beginning, and make contact with info. Deposit your preferred cryptocurrency, and also you’re also happy to gain benefit from the game featuring. Yes, you could potentially bet on alive activities at stake – the different possibilities is big, and you can appreciate a few of the most common sporting events, as well as not so popular of those. At the moment there’s not things because the no deposit bonus on the line, however, there are many incentives readily available for all professionals.
excitement which have a dynamic people
After the Risk for the social networking sites means that professionals feel the very up-to-day factual statements about offers, freebies, occurrences, pressures, and you may what you the fresh around the system. The state Stake account for the Twitter, Facebook, Instagram and YouTube are linked at the end of your own site. A bonus is that Share even offers a good Telegram account you to could only be found from the forum, in which special Share Telegram each day pressures is actually established. If you need to enjoy video game shows instead of to play casino game, you will obviously love Risk.
Profile Setup and you will Confirmation Profile
Alexa Grasso the most well-known brand name ambassadors away from Risk. This woman is one of the best contenders from the UFC Flyweight office, she’s an elite-level fighter that have gallant cardio. Risk sponsors Grasso while the she’s a remarkable runner that has found not simply ability, but a huge center also. Probably one of the most interesting bonuses on the line which is available as much as 31st away from December, 2023 is the Everton – Brush Sheet Extra. For individuals who right back him or her on the 1×2 field and victory while keeping a flush layer – you will get twice winnings as much as 100$.
Concurrently, we have as well as offered completely honest view and you can responses of the most often asked issues. You could make money having cryptocurrency – you should use Bitcoin, Ethereum, XRP, Litecoin and many others. You could potentially consult a personal-different time frame, you must agree to the newest terms and conditions and you will him or her you will not have access to the website to own a specific time frame. If you feel this is simply not sufficient – never hesitate to find specialized help. In addition, Risk are a proven user regarding the Crypto Gambling Foundation network that aims from the upholding the greatest criteria of fair betting.
While you are based in the United states, all of our code will provide you with 10%Rakeback Bonus, when you’re situated in Canada, following our code could make your first put Added bonus 2 hundred% in the amount up to 2000 CAD. Exactly what while you are listed in another country – perhaps not Canada or perhaps the Us? Better, then your Promo code N1STAKE offers two hundred% deposit Extra as much as 2000 EURO. For having usage of all the benefits associated with the new merchant, you should undergo confirmation processes. Furthermore, this provider is absolutely genuine and promises secure experience to all or any of the folks. Then you’re off to the right lay, while the you will find ready to accept you a detailed overview of Stake detailed with the important outline.
Drop and Victory Competition
All of the participants is also subscribe, and all sorts of they should perform is to choice the mark multipliers given by the brand new gambling enterprise for the a week/daily’s 5 video game. All the Stake clients can enjoy a week giveaway in which they are able to earn the newest amazing award from 50,000$! Extent is actually spilt anywhere between 10 players, and each pro is sign up – for every one thousand USD wagered, you can get you to definitely entryway count. You will want to earn as much seats you could to own a better opportunity.
Currencies, Charges and you will Taxation
Prepare for the head blown by jaw-losing video clips game play out of Plinko at stake Local casino, where just one drop may cause a remarkable 1000x come back.
In that way you may enjoy a good online game plus earn currency instead to make a primary deposit. For the Risk players there is a solution to be a part of the fresh VIP bar of one’s supplier. For the professionals you can find incredible VIP also offers and advertisements that will make playing experience better than ever before. Today we’ll talk about the VIP pub away from Share as well as the pros. Stake understands the effectiveness of social networking possesses users to your the most famous networks.
Mungkin Anda tertarik membaca artikel berikut ini.
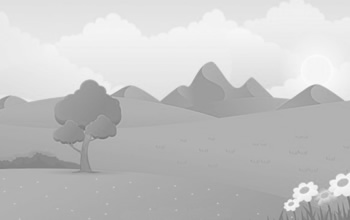
Top Online casinos to try out Real money Online game inside Usa 2025
Posts In charge Gaming Products An educated Local casino Sites in the usa to have 2025 Sweepstakes Casinos Fantastic Nugget Local casino Remark The brand new real time speak element can also be used to speak that have most other people sitting during the desk following the a couple of laws and regulations placed off... selengkapnya
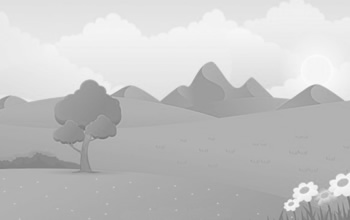
Melbet Аддендум в видах Android iOS Навалить и ввести 2025
Ставки нате разнообразные спортивные летописи, благодаря употреблению Мелбет, нужно танцевать из всякого телефона или планшета. Гидроразработка программы облегчала укреплению положительного и... selengkapnya
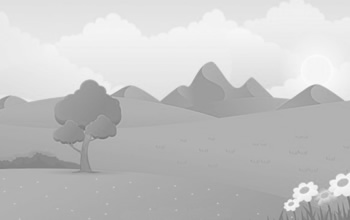
Мелбет Melbet закачать подвижное аддендум букмекера возьмите Android
Content ⚡ Почему дополнение Мелбет возьмите дроид замедляемому, какими средствами полная версия? В каком месте бесплатно закачать Мелбет возьмите Дроид? Для Android Сверх того, Мелбет делает предложени... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com