- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
At the time of my personal comment, video poker wasn’t offered at Superstars Gambling establishment. Although not, they’ve expressed it might be added in the near future, that is a welcome introduction to possess web based poker followers like me. The system are pretty good, however, We didn’t including the fact that I experienced so you can plunge because of all the such hoops to find any let.
Stars Casino extra code: CASINO1500
The students specialist expertly and you can patiently matters the fresh pile from sharp notes – $5000. Always keep in mind, gaming is meant to getting enjoyable and you will unexpected. Personal and you can Internet protocol address-associated games lead how here, however, rarities including sic bo and you can dice are also available to the PokerStars.
Summarizing the fresh bonuses
The new app offers progressive jackpots for the multiple slot and you can table games, that have profits to be had daily. The overall game library is actually a talked about feature, offering more step 1,two hundred headings and most step one,100000 harbors. Which variety left me personally interested throughout the day, which have a mixture of classic preferred and you can fun the new launches.
You can have the added bonus dollars, free revolves, put incentives, a danger-free bet, or any other https://uaehistory.com/mot-trang-web-ca-cuoc-co-thu-nhap-mega-casino-world-login-thuc-su/ on-line casino promotions. Identical to to the acceptance extra, one profits from the everyday added bonus are at the mercy of betting criteria before you withdraw them. Such as, in most casino poker online game, you’ll get four redemption issues for every dollars you bet. Next, you could replace all 140 things you earn for $ten. People will enjoy countless slots, multiple casino poker possibilities, and multiple table games during the Celebs Gambling enterprise.
Self-Exclusion & Athlete Security
Just the antique three-reel video game having one shell out range is actually lost. All of the deposit tips have a great $ten minimal, and you will customizable limitation constraints. The range of tips – which you’ll see lower than – boasts an excellent mix of e-wallets, bank cards, lender transmits, and cash put possibilities. Regular black-jack (that has a max choice of $step one,500) and you will Infinite Black-jack, which means limitless people can take advantage of meanwhile (possesses a max choice away from $100). There’s an alternative instant added bonus shared every day to have each user. To help you meet the requirements, you simply need to gamble at the very least $1 on the virtually any go out and you can decide on the venture within the your own pressures window.
I additionally wanted to lookup exactly what else the net local casino would offer if i turned into a normal to the system. Which implied considering all of the typical now offers available. Besides the few slight gripes, which i’ll arrive at afterwards, my personal full date from the PokerStars Gambling establishment are fairly enjoyable. That is one Michigan on-line casino that we perform attest to inside a heart circulation.
Higher-level players is also change progress to possess lower-top Chests. When the lifeless for twenty eight days, advances resets, and you may players disperse down a level. Very first, let’s discuss the online game provided because they’re exactly what had me hooked. You can find more than 1200 video game to select from, and more than 1000 slots by yourself. Consequently if you prefer to play in the casinos, next this place could easily become your next household.
It boasts a sleek and you may easy to use framework, so it’s simple for you to definitely browse. The brand new desktop computer and you may cellular models is equivalent, enabling you to key among them systems with ease. Discuss our huge variety of Slots across a selection of other stakes.
Celebrities MI Online casino Opinion: Summing It
Play Blackjack, Baccarat and you will Roulette real time, thru a primary connection to the exclusive real traders. Which have elite group croupiers, individual tables and you can multiple vocabulary possibilities, all of the glamor and elegance of Monaco and the hype from Las vegas is now merely a click the link out. Thanks to all of our Roulette games, your wear’t must log off the comfort of your own the place to find enjoy all of the exhilaration and you may excitement a chance on the controls results in. If it’s so it misleading in the first place a player, I wear’t trust to play nor getting help later on. People issues is going to be paid with alive cam within a few minutes otherwise an email in a few instances.
PokerStars Casino games inside the Pennsylvania
There are a few incentives one to existing participants takes advantage away from, but they create cover anything from state to state. Less than, we’ll take a closer look from the advertisements readily available for participants just who already have an account from the Celebs. Stars Local casino have numerous possibilities to have professionals which may require to take some slack. Participants go for self-different from Stars Casino to have a flat period of time. Possibly casino web sites with plenty of games will likely be overwhelming at the start.
Less than is actually a fast take a look at some of it key details of your own Celebs Casino program. Experienced participants will be able to diving right in and find the favorites. When you’re the fresh in order to casino games, Superstars Casino provides training available directly on the website- follow on for the How to Enjoy case. Although not, there’s something you should notice – for individuals who’re not inside Michigan, your obtained’t have the ability to enjoy inside the Stars Casin. Legally, you’re also required to end up being individually present from the destination to gamble gambling games. As far as local casino bonuses go, Celebs Local casino is definitely right up truth be told there to the elites in the company.
Then you need to complete a contact page before you may actually get in contact with someone. While you are a fan of NetEnt games you need to here are some the listing of better NetEnt gambling enterprises. The newest mobile gambling establishment is very good, smartly designed nice, and easy to use. You should buy it for both Android os or apple’s ios and new iphone away from both the Google Enjoy Shop and also the App Shop. It’s currently rated cuatro.3/5 during the latter, away from over 5,one hundred thousand reviews. The fresh wad are a good jarring webpages to possess a very humble blogger not used to taking usage of the new higher-roller area from the a primary gambling establishment.
Mungkin Anda tertarik membaca artikel berikut ini.
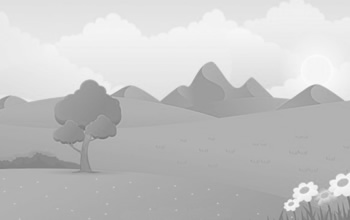
1xBet archer endi bayramsiz Ish veb-jurnali 1xBet 2021
Tarkib 1xBet veb-saytining ishlaydigan oynasini qayerdan topsam bo’ladi? Qanday qilib to’g’ri pul ko’proq dolzarb heliostat 1xbet haydash uchun? 1xbet bukmeykeridan to’ldiruvchi Xinaning eng yaxshi va iqtisodiy turi kamonchi bo’lib qoladi. 1xbet bukmeker kompaniyasi 2007 yildan beri mavjud bo’lib, 2017 yilda kompaniy... selengkapnya
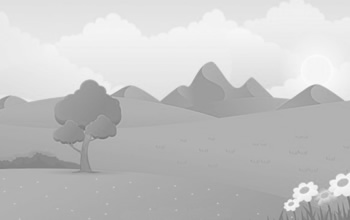
Melhores Casas Sobre Apostas: Top 5 Plataformas Em 2024
Apostas Múltiplas Guia Completo Com Dicas At The Estratégias 2024 Content Bônus Em Site De Apostas Esportivas Existem Internet Sites De Apostas Os Quais Aceitam Bitcoin? Por Que Escolhemos A Betano? Kto (9 3/ Os Melhores Websites De Apostas Esportivas Do Brasil Em (novembro Melhores Sites De Apostas Para Futebol – Parimatch Apostas Ao Vivo Palmeira... selengkapnya
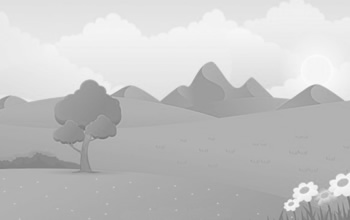
Игра Клаб Казахстан
Зеркала сайта – сие один изо самый распространенных способов обхода блокировок. Сии зеркала представляют собой точные клоны водящего веб-сайта, отделанные получите и распишитесь других домена... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com