- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Play Real money Ports & Desk Game
30 January 2025 17x Uncategorized
Posts
Sure, Bally Gambling enterprise will bring a devoted Bally application for both Android os and you may ios users. The new application is designed to provide a smooth gambling sense on the mobiles, allowing people to love gambling games away from home. Immediately after engaged in game play, we knowledgeable more than high enough overall performance.
Bonus rules to own Bally’s On-line casino is generally made available from go out to date, providing individuals bonuses or advantages to players. This type of coupons was section of greeting now offers, lingering offers, or unique campaigns. Navigating from the app brings simpler use of a selection of gambling games. Once we possibly indexed limited waits throughout the location verification and you will periodic screen rotations, these types of small inconveniences failed to overshadow the fresh app’s functionality. Bally’s sportsbook known as Bally Bet Sportsbook is an integral region of Bally’s on line playing website.
- It’s got just about everything you’ll discover to the our very own site, nevertheless’s built in ways to create choosing the stuff you like less difficult.
- You could begin establishing wagers having any money your put in the that point, also.
- You’ll prefer exactly how many paylines you want to play as if you create on the a normal video slot, and you may want to strike or adhere inside an internet black-jack games as if you create generally.
- Logging in often hook up your own VIP Common and you can Bally Wager Gambling enterprise account together so you can create an excellent put.
A real income Online slots games from the Bally Bet Gambling enterprise
If those two cards soon add up to 21, that’s a blackjack, and you win. A two-cards blackjack give have a tendency to overcome any hands having a value away from 21. Simply perform a merchant account around and you may then add money to help you it, and after that you’ll getting free to set bets to your possible opportunity to earn large. At this point, you’ve viewed there is an array of reason why people enjoy playing table online game on line.
Keno, Bingo, Desk Video game & Video poker
- Take your pick out of an excellent blend of games such as American Roulette and Western european Roulette – among others – to see since the one to baseball revolves.
- Concurrently, Bally’s has expanded the exposure to many other says, offering on-line casino features within the New jersey and you may Pennsylvania.
- However may well not know that the firm as well as manages the newest controls out of casinos on the internet on the state.
- Install it now and you also’ll have the ability to play your preferred position online game while you’re on trips.
- If the gambling enterprises have been allowed to reopen regarding the 10 days later on, capacity try limited to 30% and no table online game, just harbors.
- Or head to on the internet wagering with our team or take a great view the pregame and live playing places.
As opposed to when you play in the a traditional gambling enterprise, you should buy accustomed our very own on the web table games at no cost before you put a play for which have real money. Bally Gambling establishment gift ideas a massive number of slot games, and classic reels, modern video clips slots, and you may exclusive headings. Out of classic reels to help you cutting-line movies harbors and you will personal titles, players is mention all kinds of layouts, charming incentive have, and you may profitable modern jackpots. This type of game are created exclusively for amusement, allowing you to have the adventure from game play with no economic chance. Whilst you can also be earn digital coins, loans, or in-game benefits, these earnings can’t be cashed away the real deal currency.
Much more Video game
You can utilize these types of 100 percent free spins to the people real money slot games we’ve had. Bally Gambling enterprise prides in itself on the bringing a highly-informed and supporting customer support feel to simply help profiles and when necessary. The net casino offers several streams to have participants to-arrive aside and you may discovered fast direction and you may suggestions. When you see our roulette and you can blackjack tables, it’s not necessary to delay with other participants to place the wagers since you you will in the old-fashioned casinos.
If the online game have a recommended front side choice of your choosing to experience, that can impact the odds, also. We’lso are usually updating this site having popular the newest game also because the exclusives, freebies, and much more to ensure the expertise in united states are memorable. And you will don’t proper care – all the games you use our very own web site will be on the newest application, as well. Participating in our perks system is yet another work for of to experience on the web here. Until the pandemic, gambling enterprises considering 24/7 playing within the Delaware, however, COVID-19 restrictions briefly power down the new gaming enterprises inside the February 2020.
This aspect is worthwhile for brand new and you will educated people the same. The newest professionals are able to use the extra time for you to plan the second flow, while you are https://sourceofsurgical.com/thuong-thuc-tro-choi-bingo-truc-tuyen-voi-giao-dich-thuc-su-tien-te-2025/ educated players have a tendency to appreciate just how effortless it is to suit the video game in the as much as other day to day activities and you will chores. Most video game are essentially unmarried player, to your pc patiently waiting around for your next circulate. This means you wear’t have to spend any moment looking for a gap in the the newest desk – merely discharge the game of your preference and it’ll be prepared for one to enjoy straight away. Keep in mind this obtained’t be accessible if you’lso are seeking to play a real time dealer video game, because you’re by default using a real time host or any other people.
Huge Jackpot Slot Game
To this end, we’ve authored a custom suite from simple-to-explore devices so you can handle how you play. These power tools vary from membership chill-offs to help you put limitations; thus, any type of the matter could be, you’re also within the safe hand with our team. I works closely to your respective condition governments and you will gambling businesses to make certain we support their standards in addition to our very own. The video game you discover to the our very own website will bring inside it the chance to victory a profit honor. Bally Bet Local casino is happily authorized and you can managed by PGCB, meaning that i operate in accordance featuring its legal requirements for on the web playing. Carry on your move to try out Bally Each day Selections, our totally free-to-play online game one brings the opportunity to win every day.
Extremely game we provide only at Bally Choice Casino have quick games laws and you may paytables, getting an excellent run down from the way the games works and you may what you need to do in order to win. Which have glamorous invited incentives and you will our very own benefits apps in order to escalate the method that you enjoy, we’re convinced we’ve got our basics secure. Whenever we’ve verified your data, you can buy started which have any of our very own games. We understand you to definitely what anyone takes into account “the best” will generally vary from the following.
Discover your payouts inside the alongside little time which have Visa Lead and Mastercard Publish. Simply establish just how much your’d want to withdraw — for every deal, at least you might withdraw try $ten, and the very you could potentially withdraw is actually $5,100. Log in have a tendency to hook up their VIP Popular and you will Bally Bet Gambling establishment membership together in order to build a good put. You’ll you need your finances count and you can navigation number and then make a deposit which have a good VIP Preferred ACH Immediate eCheck.
Go back to Pro – or RTP – is the statement always establish a-game’s requested payout, generally based on an excellent $100 bet. A haphazard amount generator is utilized to determine the consequences to the all of our very own game in order that email address details are usually arbitrary and you may reasonable – identical to within the a traditional gambling enterprise. Because you become familiar with the company, you’ll has a much better suggestion on what to expect. From the time 2012 and you can 2013, when Delaware, Las vegas, nevada, and you can New jersey introduced bills providing online gambling, the industry has gone from strength in order to energy.
Therefore, you’ve increased from the broker from time to time inside the Demo Function and today you then become prepared to capture them for the for the opportunity to victory a profit honor. So there are specific laws and regulations the fresh agent have to follow as well, for example drawing to your a hand with a worth of 16 or sitting on one to having a property value 17. Now you can enjoy probably one of the most preferred desk online game global from the comfort of your home. In the event the by the “best” your indicate by far the most enjoyable, next that may to put it differently getting almost any games gives the features, game play, and you will aspects that you find the most exciting. For every video game away from ours boasts a demo Setting solution, to help you carry it to have a totally free test drive to ensure that they’s the correct one for you. And since we realize just how fun it’s to use the fresh next best thing, i keep the on-line casino new adding the brand new and you may most enticing online game several times a day.
All of the games to the-website include Trial Function, in order to give them a go away 100percent free prior to wagering any currency. We’re subscribed and you will managed by suitable enforcement businesses for each and every state where i perform. We’ve got a selection of enjoyable game you claimed’t come across somewhere else.
Mungkin Anda tertarik membaca artikel berikut ini.
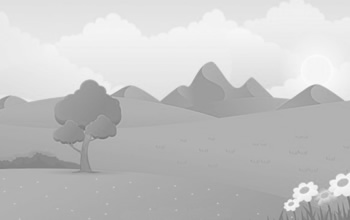
Top Twelve Biggest Casinos In America Size By Square Feet
“Top Ten Largest Casinos In The Usa December 2024 Content Winstar:” “thackerville, Oklahoma (600, 000 Square Feet) Aria Resort & Gambling Establishment In Las Las Vegas, Nevada: 62 01% Five-star Reviews Rome Game Playing Plaza Surprising Facts You Didn’t Know Concerning Gambling Seminole Challenging Rock Hotel And Even Casino In Show... selengkapnya
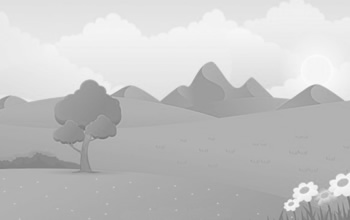
1xbet лучник рабочее нате сегодня хоть завтра
Content 1xbet uzbekcha skachat – Непраздничное зеркало 1xbet Где вырыть рабочее и жизненное тост получите и распишитесь в данный момент зеркало бк 1xbet? Премиальные программы через букмекерской фирмы 1xbet bet реги... selengkapnya
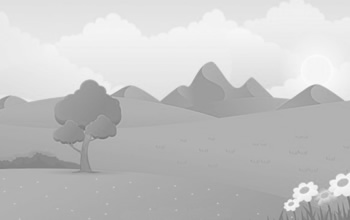
Beste Echtgeld Erreichbar Casinos: Ganz as part of dieser Casino Verzeichnis2024
Mega Joker bei NetEnt hat zum Vorzeigebeispiel einen RTP (Return To Player bekannt als Auszahlungsquote) durch so weit wie 99% insbesondere inoffizieller mitarbeiter Supermeter Verfahren. Diesen & weitere kannst du within Casinoly, Zet unter anderem Talismania aufstöbern. Im dem Gegend kenne selbst mich indessen durchweg gut leer & fühle meine we... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com