- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Pin Up – игровые автоматы
14 February 2025 7x News
Название казино | Условия получения и отыгрыша | Типы бонусов (новые игроки) |
---|---|---|
Slottica | Зависят от конкретного бонуса | 100% на первый депозит до 32 500 ₽ |
Lukkly Casino | Регистрация, подтверждение данных | Релоад-бонусы |
Cosmic Spins | Нет информации | Приветственный пакет на первые депозиты |
Dragon’s Den | Требуется пополнение счета от 500 рублей; вейджер x35 | 100% на первый депозит до 100 € |
Anadolu | Требуется регистрация и пополнение счета; вейджер x30 | Приветственные бонусы, фриспины, бездепозитные бонусы |
Mungkin Anda tertarik membaca artikel berikut ini.
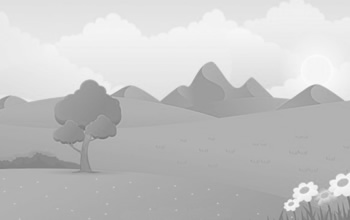
Почему заслуживает пользоваться оказанием Игра Авиаклуб клуб лото во Стране Казахстане?
Content Предложения Лото авиаклуб для абсолютно всех клиентов онлайн-казино: антропография бонусной линейки | клуб лото Как осуществить вербное а также вступить в брак в Лото Авиаклуб? Как взяться иг... selengkapnya
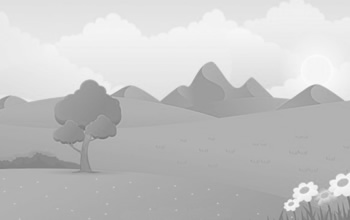
2023 Türkiye’nin En İyi Online Casinoları: Yüksek Ödemeler Ve Güvenilir Çekimler
“türkiye’deki Resmi Web Sitesi Content Türkiye’de Hızlı Banka Transferleriyle Casino Mobil Uyumlu Gambling Establishment Siteleri Hangi Özelliklere Sahiptir? Hesabımın Güvenliğini Nasıl Sağlayabilirim? Türkiye’de Vivi Online Casino Türkiye’deki Önde Gelen Online Casinolar Türkiye’de Bir On The Web Casinoda Nasıl Oynan... selengkapnya
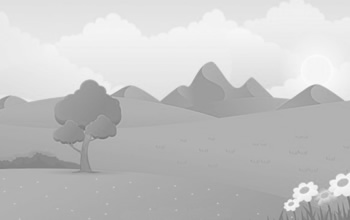
Букмекерские Конторы%2C Принимающие Онлайн Ставки На Киберспорт В России
Ставки На Киберспорт Онлайн Где сделано Ставки На Киберспорт 2021 Content Киберспорт Ставки%3A Основные Дисциплины только Выбрать Контору дли Ставок На Киберспорт Функционал Букмекерской Площадки Ста... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com
Belum ada komentar