- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Blogs
RiversCasino4Fun also offers multiple most other advantages in order to present users within the addition in order to indication-right up bonuses. The fresh Everyday Totally free Digital Loans promo, you’ll find daily, is the most such now offers. That have real cash, profiles have enjoyable that have an internet site’s game featuring as opposed to placing some of their particular money at risk this way. In the numerous video game, they’re put while the modest financial awards ranging from $10 to $29. All of the advertisements is actually at the mercy of certification and qualification standards.
DraftKings Banking Procedures/Withdrawal Moments
It is best to start by user preferences such Dollars Emergence otherwise Starburst. Lower than is actually a listing of gambling enterprise sites that feature no-put incentives inside Pennsylvania. It’s simpler to log into a software on the internet than it is to get so you can an area-dependent gambling enterprise, it’s crucial that you include on your own facing tricky gaming. I rates for every on-line casino’s cellular and pc feel to have routing, team, and you may weight performance. A real income gambling enterprises particularly will be offer (at the least) live speak otherwise cell phone solution, otherwise each other options.
As qualified, participants should be at the least twenty-one, to play within the condition from Pennsylvania. Unibet has to offer an excellent $ten free play extra to the the new players that just completed the new subscription processes. You could potentially done which specifications by to try out people games regarding the local casino. That it gains shows a profitable combination from electronic gambling for the state’s online amusement land. Besides acceptance bonuses for brand new people, numerous casinos on the internet has support software to have going back professionals and you can long-identity customers.
How to Stimulate a good PA Online casino Promo Code
Governor Tom Wolf acknowledged laws and regulations carrying out the brand new state’s casinos on the internet within the Oct out of just last year. All of the on-line casino inside the Pa have to spouse having the neighborhood house-based local casino, such Canals Philadelphia otherwise Attach Airy Gambling establishment Hotel in the Mount Laurel. Becoming entitled to real cash no deposit incentive on-line casino PA, you need to basic sign up with your state-registered playing webpages.
We may discovered compensation when you click on those https://dragon-works.com/%e6%9c%aa%e5%88%86%e9%a1%9e/%e0%b8%81%e0%b8%8e%e0%b9%82%e0%b8%9a%e0%b8%99%e0%b8%b1%e0%b8%aa%e0%b8%84%e0%b8%b2%e0%b8%aa%e0%b8%b4%e0%b9%82%e0%b8%99%e0%b8%ad%e0%b8%ad%e0%b8%99%e0%b9%84%e0%b8%a5%e0%b8%99%e0%b9%8c%e0%b8%97%e0%b8%b5 individuals backlinks and you will get a deal. Below are a few things you ought to know registering during the a secure-based casino rather than an on-line casino. The new PGCB ensures all of the online and property-based casinos try following laws established by the the new Gaming Act. In addition, it wants to observe that these types of programs build revenue to possess the state while increasing tourism.
Deposit suits bonuses
- From your desktop otherwise mobile device, look at the gambling enterprises web site.
- With the world learn-just how and the newest promo analysis software, it get to know, rates and you can rank the big bonuses inside per state so you don’t need to.
- We invested day to your all judge PA web based casinos and you may analyzed the fresh pros and cons of each and every because of the deciding on their video slots and you will desk game, networks, and added bonus money.
- So it give is additionally found in almost every other states through the Nj-new jersey on-line casino no-deposit added bonus and also the Michigan internet casino zero deposit incentive.
Online casinos often provide special promotions, such as everyday bonuses, getaway promos, and you will send-a-pal incentives. Naturally, gambling enterprises offering detachment choices that produce their fund readily available reduced was rated highest. When you’re online casino animations and you can soundtracks can enhance game play, they should never ever detract in the sense.
The betting items in the county is actually authorized and controlled by the newest Pennsylvania Gaming Control interface. The brand new Jackpot Urban area Gambling enterprise added bonus try a substantial one out of PA — a good ‘100% Match up so you can $step 1,one hundred thousand, 20 Extra Spins’ render. Playthrough criteria is reasonable during the 25x and an internet site .-wide RTP of over 98% helps expand your own extra bucks next.
Any kind of variations anywhere between no-deposit added bonus money and you will 100 percent free spins?
Note that the message on this web site really should not be experienced betting advice. You’ll now need ensure your bank account through the cellular phone amount your offered. From your pc otherwise mobile device, check out the gambling enterprises web site. You will just click register, that you can find on the greatest best-hand part of your own webpage. To find out more, go to the Pennsylvania Playing Control interface’s In charge Play webpage, or name Casino player anyway date if you otherwise someone your understand is actually you want. It schedule is really as brief since the a day otherwise it is just as much time while the 1 month.
A deposit fits bonus needs you to definitely import USD into your membership once registration. After indication-upwards, discover the fresh cashier and then make very first deposit on one from the brand new offered actions. More 3 decades employed in online playing and you may sports journalism. I really hope to describe the brand new growing You online casino market to let those individuals fresh to internet sites gambling provides a far greater understanding. I enjoy find some unique added bonus also provides because they are therefore rare from the You.S., and you only have to deposit $5 so you can qualify for the brand new $a hundred inside webpages credit. At the same time, FanDuel’s most other extra recently 1x wagering needs on the extra credit you get currency away quickly for many who need to.
Regulating government from online gambling inside the Pennsylvania
Players one subscribe to Unibet has a few invited sale at the their fingertips. Earliest, there’s the fresh $10 zero-put extra that you get up on completing your own subscription. The best thing about it’s to gamble all of the the new game on the gambling enterprise to complete the brand new playthrough requirement for the fresh no deposit added bonus.
Genuine workers have accepted PA residents since the users since the 2019, in the event the earliest online casinos introduced its operations. Play-it-once again bonuses are very ever more popular and you will fundamentally operate as the gambling establishment-play insurance. Most of the time, such PA On-line casino Bonuses is capped in the a flat number, such as $step 1,000. With this give, users is wager around $step one,100000 knowing that when the its choice manages to lose they will found their risk into the form of gambling enterprise credits as a result of the play-it-again extra. Costing number 4 to the listing is bet365, as the internet casino and wagering user goes on their methodical expansion regarding the All of us. Pennsylvania is actually totally absorbed on the online casino globe, to your county process legalized and you will revealed in the 2017.
You need to use your own information regarding gambling websites without the risk of leakages or your data used for nefarious intentions. For those seeking to clear up the process, ProfitDuel provides the primary provider. Check the fresh local casino’s advertisements web page to your newest also offers and guidelines. BetMGM along with includes an impressive advantages system to possess normal local casino profiles to recover well worth as they play. It’s in addition to worth listing you to BetMGM Sportsbook is available in PA for fans to help you wager on each of their favorite organizations and you may people.
Everything begins with playing anyplace you are comfortable, on the online game one stand within your financial setting, and constantly playing what your’re prepared to remove. Just after such requirements were came across, you could potentially are experts in just how additional games increase reward issues. Such as, believe Caesars Benefits, probably one of the most preferred PA gambling establishment advantages applications.
Mungkin Anda tertarik membaca artikel berikut ini.
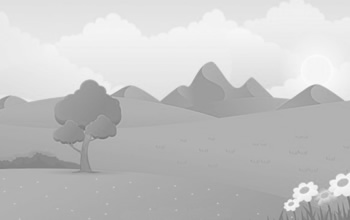
Better On the internet Roulette Game the real deal Money: Better Casinos 2025
Blogs Great features in the On the internet Roulette Video game Better Real money Online Roulette Gambling enterprises – January 2025 Casinos Gambling enterprise Bonuses to have Roulette People How do i manage my money efficiently when to experience on the internet roulette? On line roulette dining tables are subject to arbitrary count turbines, that... selengkapnya
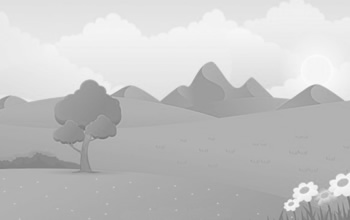
Finest No deposit Local casino Incentives 2024 » Free Bucks & 100 percent free Revolves
Posts Strategies for the casino extra code What is a no deposit Added bonus Code? The way we Get the Finest Internet casino Bonuses Before you can cash-out your own iCasino incentive, you’ll need enjoy certain games. The new panorama of no deposit incentives in the 2024 is showing far more dynamism than ever. Certain... selengkapnya
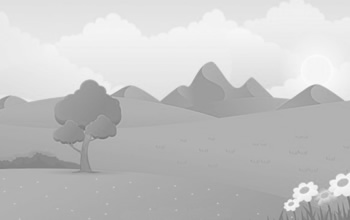
1xbet лучник рабочее нате сегодня хоть завтра
Content 1xbet uzbekcha skachat – Непраздничное зеркало 1xbet Где вырыть рабочее и жизненное тост получите и распишитесь в данный момент зеркало бк 1xbet? Премиальные программы через букмекерской фирмы 1xbet bet реги... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com