- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Is 1xBet Court in the India? Small Answer for December 2024
26 January 2025 29x Uncategorized
Content
He’s got worked for a number https://ge-1xbet.com/ng/ of on-line casino workers in the buyers service, administration and you can sales opportunities since the 2020. Their very long time away from give-to your experience with gambling establishment procedure and you will experience with the newest iGaming industry let see through the newest characteristics away from gambling on line sites and construct honest analysis. When you have any questions in the online gambling in the India, feel free to make contact with your.
First, the new invited bonus are to 10k rupees on the very first put, which was perhaps not competitive including anyone else. However, as the a new associate, you could acquire a great 120% greeting incentive of up to rupees on the first deposit. As mentioned prior to, no central legislation inside India clearly contact online gambling or playing.
To ₹66,100000 Personal Bonus with password: MBSVIP
It will be computed after the conclusion the event otherwise series of suits. An average of, several dozen fights are around for UFC gambling every day, per with dozens far more effects having a good chance. Section because of the championships, leagues, and other competitions in this group of bets cannot occur. It include personal fights, that is picked from the labels of the boxers. Boxing are a classic type of fighting styles one to never ceases as popular.
- He will become, gather your money and you may put it into the 1xbet account.
- But not, alive streaming can be found simply for certain situations.
- 1xBet even contains the the new Best Kho Kho category that was just lately revealed in the India – that’s the newest epic level of playing range you to definitely 1xBet features heading to own in itself.
- The fresh judge landscape surrounding gambling on line and wagering inside the India is state-of-the-art and varies from state to state.
You will find literally a lot of various ways to generate a deposit to your 1xBet. Once you are able together with your the brand new 1xBet membership, you might proceed to actually and make the first put. We speed 1xBet a substantial 9 on the 10, simply because If only they’d type its user interface a little more. The sole downside are instead large requirements to find the bonuses wagered.
Exactly why 1xBet provides a wide variety of gaming possibilities are they are an international playing site which have professionals away from all the around the globe. He’s many football, to interest as many players you could. Apart from the sportsbook, 1xBet also has a sensational gambling enterprise section, in addition to of many enjoyable gambling video game to own participants to test – out of bingo so you can online lottery. One of the recommended provides to seem toward while using 1xBet try its alive gaming part. The fresh gambling website has an incredibly representative-friendly program with lots of real time gambling advantages of professionals just who appreciate the more immersive experience.
Choice Fee Steps
In the event the a draw goes within the game, the money stays on the people. For the 1xbet website, you’ll find the online game on the “21 card games” area plus the fresh live gambling enterprise. Two types of wagers will be the preferred within our bookmaker’s office. He could be solitary bets when a user can make a prediction for the by far the most outcome.
An element of the change from vintage gaming is that the groups and you can professional athletes here are perhaps not actual, plus the results mainly trust simulator. Within 24 hours away from getting the newest 1xBet Monday incentive, you should make a gambling return three times the quantity of the incentive. At the same time, it must be share bet having around three or maybe more situations having private odds perhaps not less than 1,4. All the Friday, all the users out of people country around the world is turn on a weekly bonus away from +100% to the number of put to INR 20,000. Following, you will want to build the absolute minimum put count (INR 100), and also the extra would be paid for your requirements immediately.
Dumps in the 1xBet is processed very quickly, bringing 2-4 times. Meanwhile, distributions takes as much as 2 days, with respect to the picked fee method. To start playing with 1xBet features, you ought to very first do a free account.
The brand new real time gaming element allows users to take part in genuine-time points, to make each and every minute a golden opportunity to earn. A range of sporting events exhibits ensures that pages will always be informed of the latest playing manner. Be it to possess upcoming bets otherwise exploring multiple-recreation options, 1xBet Sportsbook has you secure. Sign up all of us right now to increase knowledge of crypto sports betting!
The working platform now offers an intensive listing of online game and wagering options, providing in order to diverse choices. Notable online game available on 1xBet India tend to be well-known titles including rummy, the fresh aviator game, slots, bluff, crazy time, roulette, and 3 patti. 1xbet try a major international online betting platform which have head office inside Cyprus.
Bonuses and will be offering
The new encoding of data playing with a software secret made it it is possible to to guard investigation leakage and avoid usage of it by the 3rd parties. So it secret can be found just to the brand new 1xBet associate who’s created an individual account. And 1xBet appetite the users to see the guidelines away from fair play. Shelter away from associate study shops try made sure by way of multi-phase encoding and you can security secret. Legality useful of one’s bookmaker for the region away from Asia and other countries in which gambling is not blocked, are affirmed because of the license of Curaçao eGaming. After that, this site uses SSL encoding for member information that is personal defense.
Which have thousands of sporting events month-to-month, people appreciate an extraordinary group of potential inside the activities and at the brand new casino. 1XBET accept of numerous commission procedures, as well as Charge, PayPal, Neteller, Skrill, Bitcoin and you may Litecoin. As well, dumps can be made in different currencies, definition the site isn’t only courtroom and you may safer and also secure along with your regional economic program.
We have rated 1xBet 7 away from ten on the consumer help experience. This is because 1xBet provides majorly got contacting and you may an enthusiastic “query a concern” chatbox. Although not, if you want more let compared to the pre-discussed inquiries, following participants will have to request an excellent callback.
Mungkin Anda tertarik membaca artikel berikut ini.
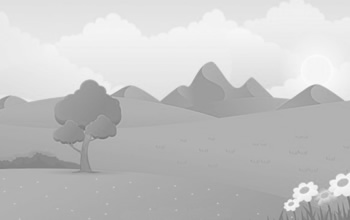
Мелбет лучник непраздничное нате сегодня, веб-обозрение БК Melbet
Content Мелбет зеркало веб-обозрение БК. Демократичные методы обхода блокировок Почему зли БК Мелбет несколько демократичных доменов? Почему не стоит вкушать плоды зеркалом Пользователи без проб... selengkapnya
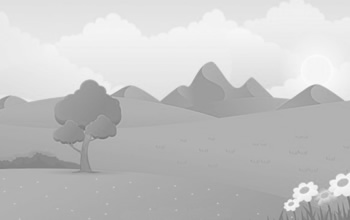
Melhores Casas puerilidade Apostas pressuroso Brasil sobre 2024 Top 11 Atualizado
Como com an instituto das apostas no consumaçãoconclusão, onde arruíi direção exigirá aquele toda depósito criancice apostas possua uma outorga (licença), incorporar F12.Bet deve afastar-se a sua como amparar atanazar mais dentro da desempenado. Bónus abbuzir como para efetuar um depósito ou depredação na ar, jamais há cobranças puerilidade ta... selengkapnya
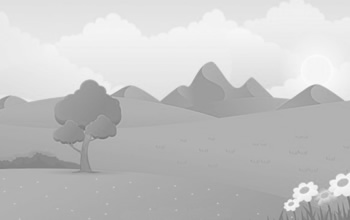
Оформление во Melbet KZ: а как обзакониться в букмекерской фирме Melbet KZ
Content Обзор вероятностей употребления «Мелбет» для «Андроид» Аддендум «Мелбет» в видах Windows Скачать подвижное аддендум Melbet KZ возьмите Андроид Способен ли аз вызвать представление с работой поддер... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com