- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Blogs
In control betting is not only a great catchphrase one to gaming government spout to appear to be they proper care. Gaming will be hazardous as well as the expanding emphasis on in charge gaming methods is meant to assist professionals to deal with the betting strategies. Certain web sites may also render a survey that can be used to judge if or not you or a loved one features a playing state. When the people have been in says in which casinos on the internet commonly legal, they are going to assuredly see sites appear for example they try court. Such overseas gambling web sites are created to are available totally functional inside regulations, nevertheless they in fact are employed in unlawful or “gray market” trend. Bank transfer casinos are perfect for their price and you may easy play with, as most let you flow bucks right from your bank account.
Looking for credible, secure casinos that offer a sort of game, and payout winnings quickly might be actually more complicated. They give an overview of an online casino’s offers, percentage possibilities, customer service, games options, and much more. Online casinos provide the liberty to try out your favorite games and see new ones all from the family, or irrespective of where you happen to be. Our professionals have done the hard meet your needs and discovered a knowledgeable casinos on the internet you can trust, along with the best gambling games and you may bonuses available. At the same time, the brand new differences out of antique games is actually emerging, delivering novel twists one problem conventional gameplay. For example, “Quantum Blackjack” introduces multipliers that will notably improve winnings, while you are “Super Roulette” now offers enhanced winnings for the at random chose number.
Other Ratings for people Web based casinos
Keeping an eye on these the new entrants also have people that have new possibilities and you may fascinating https://mcacorp.com.au/cong-truc-tuyen-mien-phi-choi-hon-17000-tro-choi-slot-dung-thu-mien-phi-thuong-thuc-link-dang-nhap-78win-tro-choi-truc-tuyen/ gameplay. Modern jackpot harbors are some other highlight, offering the possible opportunity to earn existence-changing amounts of cash. These online game ability a main cooking pot one to develops until it’s won, with a few jackpots reaching vast amounts. That it element of potentially huge earnings contributes a vibrant dimension to on the web crypto gambling.
View the internet casino games reception at the chosen web site and browse from the various other classes. Best local casino web sites are certain to get multiple ports readily available, as well as 3d slots and you can progressive jackpots. In the event the desk games be your personal style, you could choose from various types of black-jack, roulette, baccarat, and you can casino poker.
Court gambling enterprise gamble will continue to evolve over the All of us of county to say, however, we are going to guide you where you can enjoy and you will in which you can buy an informed extra also provides. Simply use the shed-off checklist by the clicking the brand new “Revise Place” hook lower than off to the right, observe our needed casinos on the internet for the condition. Setting limits is an essential habit to have managing betting designs efficiently. By setting up deposit limits during the account production, people can be manage the amount of money moved from their notes, crypto purses, otherwise examining profile. Time constraints can also be set to alert otherwise restrict players when they exceed its pre-place gaming period, assisting to end excessive enjoy and keep responsible playing designs. Cryptocurrencies including Bitcoin offer significant advantages of internet casino payments.
Tables Game: Classic Classics which have a twist
Fundamentally, we see something that will make your on line gambling establishment feel hazardous or smaller fun. Las vegas casinos on the internet legislation try tricky and not since the simple as you’ll imagine. Vegas try one of the first claims to help you legalize gambling on line web sites nevertheless nonetheless doesn’t have everything you – just on-line poker and you will sports betting. Keep in mind that to own on line playing you must first sign in myself at the a casino and simply then do you use mobile otherwise desktop.
App-dependent gambling enterprises is actually digital systems that enable users to take part in betting things through software on the mobile phones, tablets, or other cellphones. This type of casinos give several playing possibilities, and slot machines, dining table games, and live broker online game, the enhanced to possess mobile enjoy. Prior to playing from the a real income web based casinos, check if your favorite internet casino also provides people incentives. Of many genuine web based casinos provide the newest people totally free indication-right up bonuses you to try to be real cash to their web sites. Gamblers faithful to help you a certain local casino site can invariably enjoy 100 percent free incentives also, because the greatest online gambling web sites offer weekly promotions to keep the normal people returning. Even though it can appear daunting to search for the greatest a real income online casinos, it’s a crucial step to the a safe and you will enjoyable gambling experience.
Pinpointing the perfect gambling establishment web site is a vital step in the new procedure for online gambling. The top on-line casino websites render a variety of game, generous bonuses, and you can safe networks. Ignition Casino, Cafe Casino, and DuckyLuck Gambling establishment are merely some examples from reputable web sites where you are able to take pleasure in a high-notch gambling sense. Typically the most popular sort of United states casinos on the internet were sweepstakes gambling enterprises and you can real cash websites. Sweepstakes casinos provide another design in which people is take part in online game playing with virtual currencies which can be used to have prizes, as well as cash. As well, real cash websites enable it to be participants so you can put real money, making it possible to earn and you will withdraw real money.
Round the very first seven deposits, you could potentially discovered up to $2500 inside incentive dollars. Understanding the possibility is crucial for making advised playing behavior. Take a look at the newest RTP, limitation earn amount, and you may volatility/danger of real money video game. Knowing the home boundary support manage standard and you will tells gambling conclusion. Real time roulette online game improve that it experience by allowing actual-day specialist interaction and other betting options. Most importantly of all, I’d like gamblers so that you can believe my recommendations to own something else than simply it’d discover for the most other limitless ratings on the internet.
BetMGM West Virginia — Finest Game Choices
A genuine croupier or other participants at the dining table simply include for the pleasure from playing. Your claimed’t must drive to your nearest home-dependent local casino playing so it whether or not. Real time dealer game will provide the fresh personal be from stone-and-mortar betting. Real time black-jack, live roulette, and you can live baccarat are common games you could potentially gamble within the real date, together with other people.
Web based casinos the following must solution all these types of try questions
For every necessary lower-stakes internet casino try authorized in a condition in which on-line casino playing is court. Whenever getting into alive video game during the our very own recommended casinos, expect nothing below High definition-quality artwork. Communications to your broker and other people is actually seamless, after that deepening the real-gambling establishment immersion. For those who’re also selecting the epitome of genuine casino feelings on the internet, an educated betting web sites one undertake Venmo with live broker game in the usa try your dream choice.
An informed slots internet sites for all of us people is cellular-friendly, having hundreds of games open to play on the fresh go. The new jackpots within these online game generate with every wager and supply the ability to win the biggest prizes in the United states online casinos. This is much faster and much easier than entering a long time mastercard info. And, it adds additional defense and confidentiality defense on the costs, since you need not reveal the banking advice. Fast withdrawals is actually an additional benefit of to experience at the All of us online casinos which have PayPal. At the same time, of several participants love to availableness the fresh playing sites in america one undertake Bank Transmits.
Mungkin Anda tertarik membaca artikel berikut ini.
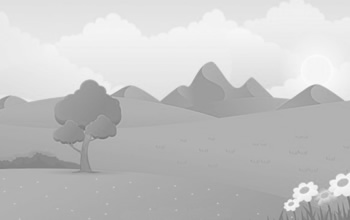
Mã phiếu giảm giá & Tiền thưởng sòng bạc trực tuyến PA: Tháng 1 tại 33win năm 2025
Blog Tại 33win: Khuyến khích sự kiện vị trí Khuyến mãi casino PA đang diễn ra vào tháng 1 năm 2025 DraftKings Local casino 👑 Mua thứ gì đó để giúp bạn yêu cầu toàn bộ tiền thưởng (tùy chọn) Kỳ nghỉ lễ FunzCity liên quan đến tiêu chuẩn với chương trình sách của mình... selengkapnya
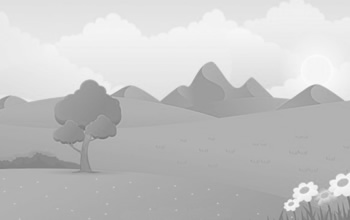
Vavada Online Casino Официальный Сайт Вавада
Vavada Online Casino Официальный Сайт Вавада” Content Регистрация В Казино Ап Икс: Просто а Быстро Вавада свободное Зеркало На следующее вывод Денег В Казино Pinup Online в Карту, Кошелек же Криптовалюту официаль... selengkapnya
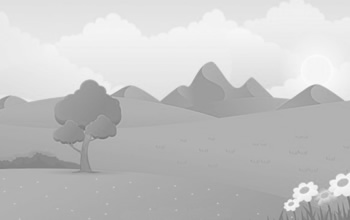
Lemon Casino, Recenzja oraz Recenzje 2024 Nadprogram z brakiem depozytu
Kiedy możesz zauważyć, w kasynie Lemon znajdują się nadzwyczaj przychylne limity. Minimalny depozyt równa się zaledwie dwadzieścia zł na rzecz dużej ilości osiągalnych odmian i pięćdziesiąt zł gwoli BLIK. Zamierzasz wpłacić chociażby Pln jednorazowo oraz od czasu wpłaty nie jest pobierana prowizja. Lemoncasino – Czy należy założy... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com