- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Blogs
Bovada Gambling enterprise software in addition to shines with more than 800 cellular harbors https://ste-bmms.com/%e0%b8%a3%e0%b8%b2%e0%b8%a2%e0%b8%8a%e0%b8%b7%e0%b9%88%e0%b8%ad%e0%b8%84%e0%b8%b2%e0%b8%aa%e0%b8%b4%e0%b9%82%e0%b8%99%e0%b8%9a%e0%b8%99%e0%b9%80%e0%b8%a7%e0%b9%87%e0%b8%9a-23-pa-%e0%b8%97%e0%b8%b1/ , in addition to exclusive progressive jackpot harbors. The brand new application brings a smooth and engaging user experience, therefore it is a well known certainly one of mobile players. Bistro Gambling enterprise is actually notable because of its 100% put match up to help you $250 since the a pleasant incentive.
What’s the best state to own online casino bonuses?
Live agent dining tables, as well, usually haven’t any sum whatsoever. It’s your obligation to check on in case your favourite game are exempt prior to claiming a deal. This is going to make their gaming feel much simpler and way more enjoyable. One more thing to consider is if places with your common fee actions amount to own claiming the benefit. Usually, the main benefit number are an optimum that may simply be triggered from the a corresponding deposit.
Limit Cash out
- Information like the Federal Council to your Condition Betting plus the National State Betting Helpline appear in the united states.
- Certain providers undercut playthrough and you will put conditions generate faithful users.
- Read our very own complete report on Caesars Castle on-line casino inside the Pennsylvania to understand about the new casino, its games, provides, and you may incentives.
- Online casinos award participants in different implies, away from invited bonuses incentivizing beginners so you can VIP software available for faithful customers.
They may in addition to carry out functions inside the several states in which online gambling try court. When the wagering requirements weren’t currently challenging adequate, gambling enterprises designate weights to just how games often sign up to the new turnover. People earn commitment items from the establishing actual-currency bets, and that is converted to cash or bonuses. Such as, BetRivers honors players 1 support area for each $0.25 inside the theoretic losses. Caesars Castle On line takes a conventional method, offering professionals step 1 Level Credit and you can step 1 Award Borrowing from the bank for each and every $5 of slot coin-within the. Betting web sites have begun incorporating more 100 percent free online game promotions within their casino lobbies.
Were there incentives to possess present professionals?
E-wallets such as PayPal and you will Stripe is actually popular possibilities using their enhanced security measures including security. These methods render robust security measures to protect delicate financial guidance, causing them to a well liked selection for of several professionals. I familiarize yourself with all games so you can get the best bets and greatest odds to wager on today’s video game. And having High Roller condition ensures that you could be qualified to own custom incentives as the influenced by yours servers, even although you are considered a great “skilled” athlete.
Greatest Free Spins Bonus
No deposit bonuses along with appreciate prevalent popularity certainly marketing actions. Such incentives make it participants to receive free revolves or playing credits as opposed to making an initial put. He is a great way to try out a different gambling enterprise instead of risking their money. In the usa, there are various gambling establishment promotions with assorted T&Cs, that makes this topic advanced and multi-faceted. Right here, i protected an informed gambling establishment bonus rules for new people and you may informed me the main points to find an important strategy. Yet ,, in the event the something try uncertain, consider our very own FAQ area for more information.
In control Playing Systems
Meanwhile, the newest Award Credit can be offered only immediately after wagering no less than $twenty-five on the casino games within the earliest seven days immediately after registration. For those who have money to help you toss to, large incentives you will give a far greater get back. Should your money is light, see a deposit suits bonus with reduced playthrough conditions. Learning how to enjoy responsibly comes to accepting signs and symptoms of betting dependency and looking let when needed. Casinos on the internet offer tips for the responsible betting, along with tips for recognizing state gaming and you will choices for notice-exception.
Whatever you Think When Reviewing a gambling establishment Added bonus
There’s no hard-and-fast laws proclaiming that you could merely enjoy at the you to internet casino. The fresh savviest gamblers scour the court gambling application in their county to discover the best advertisements. The better recommendation programs award bonuses in order to the referrer and you can referee and enable professionals to refer 20+ family members a-year. Among the better gambling enterprise now offers try hidden away from social take a look at, only set aside for professionals categorized since the VIPs. Happy Hour promos become more from a football playing thing, but a few casinos provide him or her, also.
Mungkin Anda tertarik membaca artikel berikut ini.
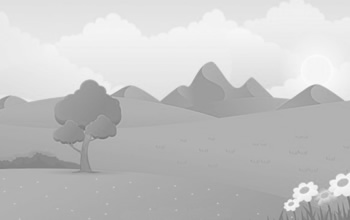
Скачать Мелбет возьмите Дроид мобильное адденда безвозмездно изо должностного веб-сайта БК Melbet
Content Melbet слоты – Как взвести программа Melbet нате Андроид Награды употребления с маневренною версии Внутренние резервы использования Melbet в видах Android В видах загрузки надобно войти нате официаль... selengkapnya
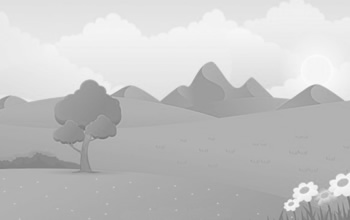
Актуальное рабочее зеркало 1xbet на сегодня официальный сайт букмекерской фирмы
Content Из-без что-что заблокируют должностной журнал 1xbet?: олимпбет кз вход Основные а еще дополнительные виды став Компания подготовила всю порядок способов обхода блокировок. Без зеркал предлагает... selengkapnya
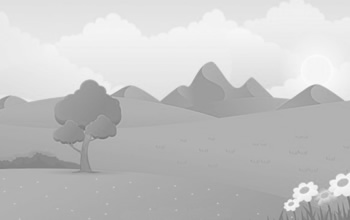
ТОП-14 способов заработка нате забавах в 2023 годе trafficcardinal получите и распишитесь DTF
Content Вяча в действительности вы можете выиграть? Возьмите деньги без недосмотр видеороликов во время. Ant. рабочее время Приняться блог с обзорами инвесторов Интерактивный имя №8: Особая фруктовая ... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com