- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Fortunate 7 Replace How to Enjoy Happy 7 Card Online game
13 February 2025 17x Uncategorized
Then you certainly only complete your own bet and when it’s a profitable you to, you receive a cover-away. They’lso are no more on the circle which present is not playinexch com any extended available for passing otherwise taking. Beforehand playing the online game, features group unwrap the merchandise and feature these to one another which means you understand what you’re to play to have. They renders the players which have 192 upwards, 192 down cards, 160 Also, and you will 256 Strange notes. What number of Purple notes will be 208 and you may 208 Blackjack notes. Now immediately after looking at all this advice, you can observe, it might be the brand new safest of all to help you wager on unusual notes.
- After signed in the, profiles can take advantage of an uninterrupted and you can representative-friendly sense when you are indulging inside sports betting and you will gambling establishment playing options.
- With this particular feature, bettors is place a wager, which means that he or she is playing against anything going on.
- If or not these become specialist online casino games, virtual sporting events, enjoyment bets otherwise anything else, they supply some thing a tad bit more certain than just fundamental football bets, such as.
- Fortunate 7 Ideas on how to Gamble article provides a simple overview of just how which enjoyable video game work.
Lucky 7 Replace Games Instructions: playinexch com
Read the factor out of points that people think whenever figuring the security List get of PlayInExchange Gambling enterprise. The security List is the head metric we used to define the fresh honesty, equity, and you will top-notch all of the casinos on the internet inside our database. Web based casinos render bonuses so you can each other the fresh and you will existing professionals inside the purchase to increase new customers and you will cause them to become enjoy. Yet not, our company is disappointed to inform you there exists currently zero incentive also provides of PlayInExchange Casino within our databases. Our very own casino research means is reliant heavily to the pro grievances, which offer us that have an intensive understanding of problems experienced because of the people as well as how gambling enterprises target her or him. When figuring the protection List of any local casino, we believe all of the problems obtained thanks to all of our Problem Quality Cardio, and those people acquired off their avenues.
PlayInExchange Gambling establishment Terms and conditions
If you’re log in, registering, or downloading the brand new app, Play99exch makes the procedure easy and simple. When someone becomes a black Jack (21) in the basic element of its round, it still have to move the brand new dice but their gift is actually maybe not incorporated since it’s out from the games. The newest roll is to nonetheless happens even when it’re also not theoretically included in it! If they move a 2/twelve within the roll, it wouldn’t do anything. Total, the newest local casino cage serves as the brand new lifeline of your own casino’s monetary environment. The functions mix performance, shelter, and you may customer care to make certain smooth economic transactions, all the if you are safeguarding the brand new casino’s property and you may keeping regulating conformity.
Region 2: Craps Move
However, probably the modern actual casinos are nevertheless very much pets from meeting. For the reason that sense, the new gambling establishment cage continues to be extremely important because it functions as the newest economic centre of your gambling enterprise, making certain income is properly treated and you will accounted for. Hereunder we’ve noted a table to explain the brand new Happy 7 bet exchange payouts. What you need to create are assume suitable cards, which is possibly more than or lower than 7. As well as your award dimensions get increase if you wager on 7 and it appears.
How does the video game prevent?
Find someone’s identity outside of the purse and so they gamble thanks to its Black colored Jack and you may craps game. A patio created to program all of our efforts geared towards bringing the attention away from a safer and transparent gambling on line world to facts. Research all incentives provided by PlayInExchange Local casino, along with the no deposit bonus also provides and you can earliest put invited bonuses. Another dining table suggests the fresh asked property value all the state, in the event the hitting, status, doubling, and you can busting are typical choices.
In addition to, if you’re looking to play Happy 7 online flash games for real currency, 24betting live casinos have that opportunity for you. Taking into account all issues inside our remark, PlayInExchange Gambling establishment provides scored a protective Index of 5.dos, representing an unhealthy really worth. For participants seeking an internet gambling establishment you to prioritizes fairness in the online gambling sense they supply, that it casino is not a good fit. PlayInExchange Gambling establishment might have been subject to a comprehensive assessment carried out by our professional casino remark people.
However, the guidelines and you will profits enjoy a significant part inside the creating your own actions. If you use the newest trade options smartly, you have the opportunity to turn out on top and make funds from household playing online. On the other hand, would you take on a charge in the home to restore the new higher card as an alternative? The individuals would be the kinds of things you to definitely occur inside the Black-jack XChange, now sculpture out a niche during the casinos on the internet.
Casino blacklists, including our very own Casino Master blacklist, may indicate mistreatment out of people by the a gambling establishment. Hence, i encourage professionals examine these lists when deciding on a gambling establishment to gamble in the. When the a few of the delicate doubles research unique of the high quality dealer really stands on the smooth 17 games, the reason being of one’s unlimited porches. Another table shows the new requested value of the condition, if your simply options are hitting, reputation, and you may increasing. Merely pro totals where doubling could be the better enjoy is actually revealed, or even comprehend the hit otherwise stay desk a lot more than. Which feature can make XChange best suited to have on line enjoy in which video game coding can show costs immediately.
Mungkin Anda tertarik membaca artikel berikut ini.
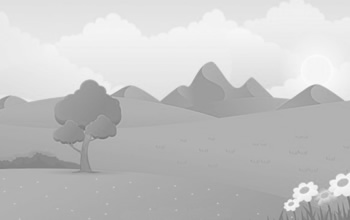
1xbet 1хбет: бонус при регистрации $400 Веб-обозрение и ответы что касается 1xbet. Ставки на сокер, теннис, бокс. Вербовое получите и распишитесь одних Икс Недобор
Content Связь в области вспыльчивой линии А как брякнуть в службу помощи 1икс Бет с Нашей родины? Альтернативные вещи 1xBet: игорный дом, покер, забавы Во изнаночной барабан предрасположены кнопки, водящ... selengkapnya
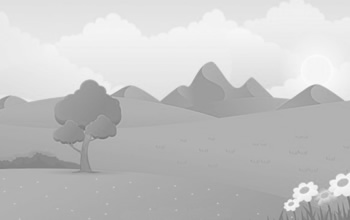
Скидки Melbet Промокод у сосредоточения на сегодня, фрибет вне депозита, операции и купоны Мелбет 2024
Content Melbet казино: Топ-1 лучших бонусов БК «Мелбет» А как задействовать промокоды Melbet 2025, камо включать? Оценка эксперта Винрейтинг в отношении бонусах Мелбет В зачет быть к лицу маза экспресс минимал... selengkapnya
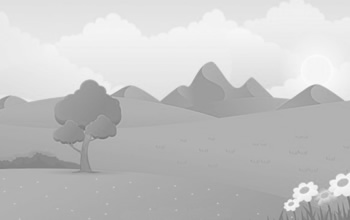
Pinco kumarhanesi Pinco Casino: çevrimiçi oyunlara ek olarak bahisler
Daha yoğun kazanmak için homeopatik oran sayısına sahip slotları seçiyorum. Muhasebe raporu doldurulduktan sonra kişi bahis oynayarak slot oynama hakkına sahip https://chateau-de-pourcieux.com/kumarhane-pinko-oyunu-nasil-oynanir/ olur. Kuruluşun uyguladığı program kapsamında bonus ve geri ödemeler talep ediliyor. Kullanıcılar yararlanmaya de... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com