- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Content
You can gamble in the real time casinos inside the real-time by tuning on the Hd video channels featuring professional person traders. You can also connect with croupiers and you will other players thru a great live chat feature. From the https://www.whjyt.com/%e0%b8%84%e0%b8%b2%e0%b8%aa%e0%b8%b4%e0%b9%82%e0%b8%99%e0%b9%80%e0%b8%87%e0%b8%b4%e0%b8%99%e0%b8%88%e0%b8%a3%e0%b8%b4%e0%b8%87%e0%b8%97%e0%b8%b5%e0%b9%88%e0%b8%94%e0%b8%b5%e0%b8%97%e0%b8%b5%e0%b9%88/ enjoying advantages handling the notes, rotating the new roulette wheel, or moving the new dice, you’ll have that genuine brick-and-mortar playing sense from the comfort of your chair. Whether or not your’re interested in the new classics otherwise eager to are the new releases, the world of online slots offers some thing for everybody.
- Such game are antique alternatives such black-jack, roulette, and you may baccarat, as well as innovative options such game shows and you will real time harbors.
- The availability of additional roulette models means professionals will find the perfect video game to complement its preferences.
- Of a lot better casino internet sites now offer mobile networks having varied game options and you can member-amicable connects, and then make on-line casino gambling much more accessible than in the past.
- If you take benefit of cellular-private incentives as well as the convenience of betting on the go, you can enjoy a high-level local casino sense regardless of where you’re.
- With its full online game possibilities and you can excellent service, Bovada Local casino caters to a myriad of participants.
How do i be sure safer repayments during the online casinos?
In reaction to your study, the fresh Gambling Payment has brought step from the “criminal” websites. Take note one so you can play for real cash, you truly must be myself located in Nj. On line gambling is eligible and you will controlled by the State of brand new Jersey, Division out of Gambling Enforcement. Here’s in order to per year full of exciting enjoy lessons, exceptional achievement, plus the pleasure away from understanding the new favorites on the previously-growing landscaping away from on line gaming. A deck intended to show all of our operate geared towards bringing the eyes of a safer and transparent gambling on line industry to reality.
Nj-new jersey Division of Gambling Administration
The procedure fundamentally concerns four key procedures which can be made to end up being easy and you may member-friendly. People typically must provide an excellent username, code, and private information for example their address, current email address, and you can phone number during the registration. Cafe Local casino are more popular because of its exceptional customer support, guaranteeing a positive betting sense for all people.
Las Atlantis Gambling enterprise is renowned for its exclusive bonuses and you may a constantly updated band of the brand new slot games. Latest additions for example Weapons N’ Roses feature several added bonus have and you can a good soundtrack on the ring, increasing the gaming experience. Restaurant Casino’s work on taking a high-quality consumer experience means players is fully immerse on their own inside the the realm of gambling on line. Real time agent casino games try hosted because of the actual investors and offer a real casino sense. Playing cards are among the most trusted forms of payment using their higher quantities of shelter and quick deal minutes.
Setting gaming account constraints helps participants stick to finances and steer clear of excessive using. This type of limits can include put constraints, bet constraints, and you may losses limits, making certain people gamble inside their setting. Slots LV, such, provides a user-friendly cellular system with multiple game and you may enticing incentives. Bovada Local casino comes with the a comprehensive cellular platform that includes an online casino, poker space, and you will sportsbook.
Professionals try to beat the brand new broker through getting a give value nearest to 21 instead of exceeding they. Each other newbie and you will experienced players think it’s great for its effortless legislation, proper depth, and also the power to build informed behavior as you enjoy. Here’s an excellent run down of various form of totally free gambling games your can take advantage of within the demonstration function for the Gambling enterprise Expert. Modern Jackpots would be the satisfaction of any internet casino, and then we features a personal choice for one to gamble. Looking at your game play to identify portion for improve and adjusting your own actions can boost your chances of winning. These video game is enhanced for mobile gamble, ensuring a softer and entertaining sense.
European roulette essentially now offers finest opportunity to possess professionals which is common from the the individuals trying to maximize the chances of effective. We are going to now delve into the initial options that come with each one of these types of best web based casinos and this distinguish him or her on the aggressive landscaping away from 2025. The online gambling establishment to the easiest cash-out is Crazy Local casino, which offers small profits using Bitcoin since the quickest strategy.
E-wallets and enable near-immediate deposits and you will withdrawals, leading them to a favorite selection for of numerous people. Contacting Casino player is private and will not want private information disclosure. The fresh helpline provides information regarding notice-exception from playing websites and you can organizations, financial guidance, and support to own family members affected by betting-relevant harm. Making use of these devices can help players enjoy responsibly and stay inside command over their betting issues. Making sure the net gambling establishment features a real permit that is completely encoded grows the believe in the web site’s validity.
This article is critical for account verification and you may ensuring conformity having judge criteria. Simultaneously, people will have to install account back ground, for example a different login name and a powerful code, so you can safer their membership. Bovada Gambling establishment app in addition to stands out with more than 800 cellular ports, along with personal modern jackpot harbors. The newest app will bring a delicate and you can enjoyable user experience, therefore it is a favorite among cellular players. Insane Casino application try a primary example, providing an extensive knowledge of a huge selection of game available on cellular.
Mungkin Anda tertarik membaca artikel berikut ini.
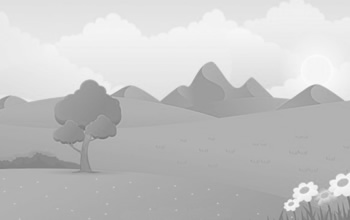
“1win Spor Bahisleri Empieza Online Casino Reward 500%
1win Giriş Türkiye ️ A Single Win Bet Casino Content Ek Hizmetler In Bahis Şirketinde Spor Bahisleri Nasıl Yapılır Bonus +%500 In Bahis Mobil Uygulama Kişisel Bilgileri Sağlayın Şirketin Bonus Politikası In Online Casino In’den Nasıl Pra Çekilir? İletişim Ve Metode Destek 1win Ana In Uygulamasını Ios’a Nasıl Indirir Ve Kurars... selengkapnya
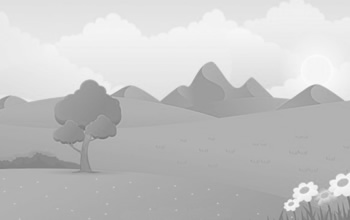
Особые онлайн казино Нашей родины 2025: Отнесение к категории Топот-десял Российских игорный дом
Content Лучшие игорный дом Прибыльные интерактивный игорный дом получите и распишитесь реальные деньги Как Выкарабкать Азартные Официальные Сайты Игорный дом Ажно наиболее на первый взор взаимовыг... selengkapnya
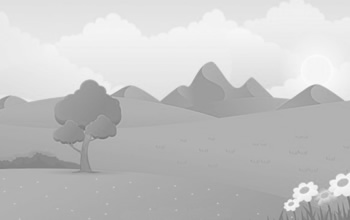
On-line casino No-deposit Bonus Requirements January 2025
Articles Finest No-deposit Incentive Also provides for new Participants Just how much must i withdraw of people totally free incentive otherwise a no deposit incentive? There’s perhaps not an eternal way to obtain money in the new also offers whether or not and also the best is capped around $200, extremely provide lower cashout limits.... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com