- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Compañía De Apuestas Apuestas Deportivas Online 1xbet ᐉ 1xbet Com
4 February 2025 23x Uncategorized
Online Sports Betting At 1xbet ᐉ Mobil 1xbet Com
Content
- 🌟 What Should A Player Do If These People Lose The Search Engines Authenticator Key Regarding 1xbet?
- Games
- Faq On Authorization In 1xbet
- Step A Single: Go To The Particular Homepage Of 1xbet Pk
- Bet Login Around The Official Website
- What Are The Advantages Of Bets Live?
- Something To Be Able To Keep In Thoughts Before Placing Reside Bet On Sports
- Casa Para Apuestas 1xbet — Apuestas Deportivas En Línea
- Sports Betting
- ⚽¿en Qué Deportes Sumado A Eventos Se Puede Apostar Con 1xbet?
- Casino En Directo
- What Are Live Bets?
- Bet Affiliate Marketer Program
- 🔒 Where Is The Player’s 1xbet Account Located?
- Casino Ao Vivo
- Live Bets – In-play Betting On Sports
- How In Order To Enter 1xbet In The Event The Official Site Is Usually Inaccessible?
- Available Bet Types
- 💰how Can You Earn Money With 1xbet? Predictions On Athletics Events
- 🥇 شركة المراهنات 1xbet — ما الذي تريد معرفته؟
- 🛡️ May A Person From Pakistan Change Their Files Within The 1xbet Consideration?
- Main Features Regarding The Player’s Personal Account
- Client Account Blocking
- Promo Code
- Société De Paris 1xbet – Paris Sportifs En Ligne
- 💰comment Pouvez-vous Gagner De L’argent Avec 1xbet? Sobre Faisant Des Pronostics Sur Des Événements Sportifs
- Setting Up 2 Factor Authentication Within 1xbet
- Casino En Direct
- 💰¿cómo Puede Ganar Fortuna Con 1xbet? Pronósticos De Eventos Deportivos
The almost all common reason intended for being unable to log in to the account will be incorrectly entered files. This usually happens when the end user has chosen typically the wrong language type, accidentally pressed the Caps Lock key, or confused the sequence of amounts in the pass word. To resolve the issue, carefully enter the particular login credentials once again. This way, the bookmaker tries” “to guard its clients’ company accounts from fraudulent pursuits and hacking. The player will want to enter a new captcha and confirm their login in order to their account. It is also possible to generate an bank account at the bets site from a smartphone or tablet, either in some sort of browser or throughout an app that could be downloaded and installed without registration.
Fans of gambling is not going to languish in holding out and catch the broadcast with typically the announcement of successful numbers. A distinct section offers a wide selection regarding slots, card and other scratch cards (including live dealers), as well as lotteries, bingo, crash online games and” “etc. At 1xBet, users are given accurately those free wagers which might be needed simply by a particular player. The user decides for which sport and with just what conditions the promotional code will be valid.
🌟 What Should A Gamer Do If These People Lose The Yahoo Authenticator Key For 1xbet?
The customer himself chooses some sort of poker table dependent on his choices to play Hold’em or Omaha. Tennis in the series is represented by simply greater than a hundred events on any presented day. This is usually especially valuable, due to the fact tennis draws are held, at best, 2-3 days throughout advance. We control to achieve satisfactory depth of typically the line due to be able to the fact that will we offer to be able to bet not only on ATP and WTA tours, but also on Challengers and ITF occasions 1xbet ng.
- After producing an account, verifying and making your first deposit, you will certainly receive the same sum of money from the administration to your own bonus account, supplied that it is far from more than 6000 PHP.
- For NHL matches, the lineup is so thorough that it is usually comparable to the football one.
- With typically the help of typically the mobile client, typically the user gains immediate access from their smartphone to typically the full range involving products and services offered by the bookmaker.
- Periodically, specialists involving the gaming internet platform perform specialized work, which will will take place at night time.
It often happens that will the problem along with accessing the company’s website, and eventually, failures in the particular authentication process, may well be caused by insufficient quality of the internet traffic. This” “issue needs to end up being addressed by altering the settings of the proxy hardware, which may become overloaded. If generally there are interruptions with all the internet signal or even its unstable procedure, the user need to contact the provider to resolve such problems. We’re constantly increasing our applications in addition to use all the capabilities of recent mobile devices. Our key aim is in order to give you the ultimate end user experience, alongside convenience and security.”
Games
To determine the legality associated with playing in the betting shop or online casino, check typically the nuances of local legislation. To do this, in a new convenient browser, enter the name involving the bookmaker’s workplace, after which an individual just need to click on the particular link to insert the betting program. The player is going to be directed to the particular starting page regarding the website, in which they can watch top matches or even proceed directly to loading their user profile. There’s no want to head out there within the search with regard to a betting go shopping to place your current bets.
- There’s no need to squander time searching with regard to web platforms where mobile applications may be available.
- On neutral forums, players often focus on the problems and shortcomings of the betting clubs rapid something that the companies themselves try certainly not to mention.
- Especially for those who favor to place athletics bets and play slots from their particular phones, the leading online operator gives the use regarding its proprietary application.
- An independent study of comments on thematic platforms shows that 1xBet is not often complained about, if.
- 1xBet seemed to be founded in 2007 and in recent times has become a single of the world’s leading betting organizations.
The company provides developed original mobile phone applications suitable intended for Android devices in addition to iPhones. With the help of the mobile client, typically the user gains primary access from their own smartphone to typically the full range involving products and services offered by the bookmaker. Besides sports betting and visiting on-line casinos, clients also can bet on the weather, play TV SET games, online online poker, and online bingo.
Faq In Authorization In 1xbet
We offer betting on major competitions in all major exercises, not merely CS, LoL Dota 2, although also, such as, Valorant or FIFA. The company already presents odds on a lot more than ten different disciplines at any kind of given time. In addition to sporting activities betting itself, 1xBet also accepts wagers on non-sports activities, for example, the next thunderstorm at airports all over the world, election results, the winner of audio competitions or motion picture awards. The main plus of reside betting is that you can succeed big in a short period of period. The player decides the account currency at the moment of enrollment and will not end up being able to alter it. When mailing money coming from a conditional dollar account in order to details within foreign currency, check in enhance whether such a transaction will move with automatic alteration – that will be, whether it will take location at all.
- Every customer enjoys making predictions on matches enjoyed by their favorite team.
- In the second case, the online games undergo a exclusive certification proving that the determination in the winner does not allow interference in the process from the casino staff.
- To increase the profitability of the wager, make a gamble with an show of two or more events, however in this case, typically the bet will be played as long as just about all the predictions incorporated into it come correct.
- Virtual sports are also not forgotten, but it is fundamentally various.
- However, its not all players would like to burden their particular smartphone memory with unnecessary software.
- Keno draws plus similar games usually are presented at 1xBet Philippines online casino throughout the format regarding video broadcasts.
Having your own account allows any Pakistaner gambler to generate deposits, place bets about any sports, perform slots, and guess in online casino games. An authorized player may easily participate throughout the company’s marketing promotions and giveaways, while well as work with promo codes plus accumulate loyalty points. We will discuss in more details inside the article the various ways to be able to log in to 1xBet and exactly how to avoid achievable problems with launching the account.
Step One: Go To The Particular Homepage Of 1xbet Pk
You can even bet on youth warm and friendly tournaments in typically the off-season, not to be able to mention official nationwide championships. Virtual athletics are also not really forgotten, but that is fundamentally distinct. Take into bank account that an ideal complements the winner is decided only by some sort of random number generator, and any similarities with real clubs and athletes carry out not affect something. The total number of disciplines with recommended events exceeds 60. The basis will be classic sports (football, tennis, basketball, snow hockey, as well as other” “disciplines), but the supervision also provides a good cyber sporting activities line (Counter-Strike, Dota 2, League associated with Legends, and others).
- A huge advantage of 1xBet Philippines over its competitors is the availability of movie broadcasts, even when not for just about all scheduled events, however for a very huge percentage.
- Poker, blackjack, baccarat, different roulette games” “and even craps are presented in the institution in a number of variations regarding rules.
- An authorized player can easily easily participate within the company’s special offers and giveaways, since well as work with promo codes plus accumulate loyalty factors.
- There’s no need to sit all-around pondering and evaluating up your alternatives, simply get the bets in whilst the action is unfolding!
- Every registered player in the betting business 1xBet has the alternative of two-factor authentication.
- 1xBet has made that possible to play not only about PCs, but in addition on smartphones or perhaps tablets.
With 1xBet Philippines, you get access to a thorough suite of companies tailored to fulfill your betting requires. Enjoy seamless navigation, competitive odds, a vast selection of sporting activities markets, exhilarating are living betting options, plus generous promotions. Whether you are some sort of sports enthusiast, keen on casino games, or possibly a thrill-seeker looking with regard to new adventures, 1xBet offers an all-inclusive betting experience that will always keep a person engaged and amused.
Bet Login Around The Official Website
The principle of authorization depends on typically the method of registration. To familiarise yourself together with the current offers, visit the ‘Promos’ section of the particular 1xBet Philippines website, as well as the main long term bonuses with the long validity period of time are summarised inside the table listed below. To access typically the login page, the” “end user needs to click on the “1xBet login” button around the company’s website, positioned in the top appropriate corner. After that, the web resource method will display the documentation form on the screen. Use some sort of VPN to bypass the blockage, or look for the current mirror web site, one example is, in the social networks from the administration.
- This will be especially valuable, considering that tennis draws will be held, at finest, 2-3 days in advance.
- The list of the top matches can easily include more as compared to a thousand marketplaces thanks to wagering on statistics – how many cards is going to be played, how the goal can be scored and so forth.
- We will go over in more fine detail inside the article the various ways to be able to log in in order to 1xBet and how to avoid probable problems with loading the account.
- Tennis in the series is represented simply by more than a hundred situations on any offered day.
- The most favored type of bet in 1xBet is the single bet, when the user brings only one occasion to the discount.
If the table online game is implemented inside the form associated with a graphical user interface, the winner is definitely determined only by way of a random number generator. 1xBet Philippines lineup is aimed at making it possible to predict almost anything. After producing an account, confirming and making a deposit, you may receive the same sum of money from the administration to your own bonus account, provided that not necessarily more than 6000 PHP. The gift should not be withdrawn immediately, and the winnings received because of it must be wagered. Hurry up to fulfill the particular wagering requirements, normally the bonus may be cancelled.
What Are Usually The Benefits Of Gambling Live?
Periodically, specialists regarding the gaming net platform execute technological work, which in turn requires place at night. If a player encounters a notice about technical operate when accessing typically the betting platform, that is worth waiting for their completion and then reloading the bookmaker’s website. Every customer enjoys making predictions on matches played out by their favourite team. By combining their unique knowledge using reliable statistics, consumers can change their estimations into money. They may easily” “consider the probability of just one outcome or one more, make their predictions, produce a wager slip. What’s a lot more, the 1xBet website offers customers the chance to create a winning combination and promote their bet fall with their pals.
- We offer gambling on major contests in most major disciplines, not only CS, LoL Dota 2, yet also, one example is, Valorant or FIFA.
- We adhere to typically the principle of sincerity and honesty throughout relations with our clients, so we will definitely satisfy all the commitments stipulated in the particular user agreement.
- The government schedules the main countrywide championships and international tournaments, giving estimates not only within the outcome of the meetings, but likewise on statistical indications.
- The predominant part of the collection is offered for both pre-match and live betting.
You can easily bet live in football, ice handbags, biathlon, baseball, boxing, table tennis, snooker, biking, water polo plus many other sports. A huge benefit of 1xBet Philippines above its competitors could be the availability of video broadcasts, even in case not for most scheduled events, however for a very big percentage. Even if there is no TV picture, the person can get a great idea of how the particular game is building thanks to thorough statistics both regarding previous meetings involving opponents as well as the current match. For NHL matches, the collection is so thorough that it is usually comparable to the football one.
Something To Be Able To Keep In Head Before Placing A Reside Bet On Sports
The Curacao license, under that this betting site operates, is relevant regarding all countries where there is simply no strict regulatory insurance plan, nevertheless the site has a amount of other local licenses. There is also the option of placing a are living bet just prior to the end of the match or opposition, which greatly raises your chances associated with winning. You could see which way the flow involving play is converting, analyze the action, and then help make your prediction by simply placing a gamble in-play, none involving which can be possible with a pre-match bet. There tend to be more compared to a thousand activities in our RESIDE section every time – both well-known contests and occasions for sophisticated” “sports fans.
- To familiarise yourself with the current gives, visit the ‘Promos’ section of the 1xBet Philippines internet site, plus the main extensive bonuses with the long validity period of time are summarised in the table listed below.
- The administration has provided numerous bonuses, regularly holds promotions and prize draws, and allows active customers to acquire free gambling bets to their liking.
- Enjoy seamless navigation, competitive odds, the vast number of sports activities markets, exhilarating survive betting options, plus generous promotions.
- A player will have a lot better probability of winning a bet if their prediction is built based on the previously seen part associated with the confrontation, if it is possible to identify clearly visible trends.
The 1xBet Philippines software can be accessed directly in the mobile browser. The display of elements adapts towards the sizing of the display and acquires a vertical orientation. All” “the particular functions of typically the full-screen site will be retained in the particular mobile version.
Casa Para Apuestas 1xbet Rapid Apuestas Deportivas Sobre Línea
The most widely used type of bet from 1xBet is the single bet, whenever the user provides only one event to the coupon. To increase the profitability in the guess, make a guess with an express of two or more events, in this case, the particular bet will become played as long as most the predictions contained in it come real. By creating an account on a single system, a user can easily log in through any device. There is not a need in order to re-register in the mobile app when you have a PC account. Just look for the ‘Login’ button in the interface and get into your username and even password, or authorise via social networks.
- After accumulating an adequate amount of points, they can be exchanged for some sort of promo code, which usually gives the justification to help to make a free gamble with certain situations.
- The company already presents odds on more than ten distinct disciplines at any kind of given time.
- Experienced punters can make critical money from their live bets, while beginners can count on their individual luck.
- The player will will need to enter some sort of captcha and validate their login to their account.
- The main plus of live betting is of which you can earn big within a short period of moment.
An avid gambler from Pakistan that has successfully registered plus opened an consideration using the betting company 1xBet must go through authorization to be able to access the operator’s services. The chance to log in to 1xBet Pakistan is definitely provided to customers in the company on the official site, and it can easily also be done by a smartphone throughout its lite edition and in the particular original application. Immediately after loading their particular 1xBet account, typically the player gains entry to all the bookmaker’s products.
Sports Betting
If you have a problem or question, you don’t have to wait for a great available support operator. A large area with detailed answers to frequently inquired questions will support you discover the response or solution oneself, spending minimum time. We stick to typically the principle of honesty and honesty within relations with each of our clients, so we will definitely fulfil all the requirements stipulated in the user agreement.
- Regardless with the behavior of the gamers attracted by the particular 1xBet partner, this individual himself cannot go into a without.
- The wagering club’s margin with regard to betting on top rated matches does not really exceed 3%, so that users may count on the most favourable odds.
- The client with the company will become able to safeguard their account plus gaming account coming from hacking and unauthorized use by businesses.
- We deal with to achieve enough depth of typically the line due to the fact that will we offer in order to bet not just on ATP plus WTA tours, yet also on Challengers and ITF situations.
However, not all players need to burden their smartphone memory along with unnecessary software. For such cases, typically the leading online agent 1xBet offers to utilize a compressed copy with the official website. 1xBet has made it possible to play not only in PCs, but in addition on smartphones or perhaps tablets. All you need can be a gadget and an internet relationship; slots and reside dealer broadcasts may be launched proper in the device’s standard browser! Functionally, both the ways associated with accessing the online casino do not change, except that typically the software saves some sort of bit of traffic and works as a mirror. Sometimes the official site from the bookmaker organization might be undergoing modernization.
⚽¿en Qué Deportes Con Eventos Se Puede Apostar Con 1xbet?
The bookmaker may definitely carry out verification by looking at the questionnaire data with what is written in the documents. The quality with the free wager exchanged for a promo code is usually limited. To ensure the program performs correctly, the gamer should familiarize by themselves with the minimal system requirements for that device. Even for those who have never been to a betting go shopping and do not know significantly about odds plus markets, you may figure it in a few moments.
- It is also achievable to create an bank account at the betting site from a smartphone or pill, either in the browser or inside an app that may be downloaded and mounted without registration.
- Our primary aim is to be able to give the ultimate customer experience, alongside ease and security.”
- By creating a good account using one system, a user could log in through any device.
- The previous step in the authorization process throughout the mobile client is entering the confirmation code.
- You can even gamble on youth warm and friendly tournaments in typically the off-season, not in order to mention official countrywide championships.
The final step in the authorization process through the mobile client is definitely entering the confirmation code. This blend is automatically submitted a message to be able to the player’s telephone number. Logging in via the 1xBet identifier allows for additional protection regarding player accounts in addition to prevents using client accounts by hacker.
Casino En Directo
You could bet live about sports and hit the jackpot on the web on the 1-x-bet. com website. The menu is simple to use, so you can opt for the most convenient repayment means for you in addition to peruse the broad selection of market segments on all the most popular activities across a wide variety of athletics. Neither money nor users’ personal information should fall in to third hands, which usually is why 1xBet carefully encrypts the particular transmitted data. It is impossible to be able to hack into typically the account of the particular company, unless you notify someone your password. Keno draws and similar games are usually presented at 1xBet Philippines casinos in the format of video broadcasts.
- To do this, you have to open the area with software advancements, choose the correct software option centered on the type of operating system used on the device, plus then download the particular installation file.
- Our company schedules the very best national crickinfo championships and intercontinental matches, providing respectable line fills and even a good selection involving markets.
- There will be more compared to a thousand situations in our RESIDE section every day – both popular contests and occasions for sophisticated” “sports fans.
- For such cases, typically the leading online user 1xBet offers to utilize a compressed copy in the official website.
“1xBet is a distinguished bookmaker and on-line casino with a historical past spanning almost a couple of decades. The betting club’s margin intended for betting on top matches does not really exceed 3%, thus that users can count on typically the most favourable odds. Especially for individuals who like to place sports activities bets and participate in slots from their particular phones, the top online operator provides the use associated with its proprietary computer software.
What Are Live Gambling Bets?
Join us right now and elevate your gaming to new heights with each of our top-notch services. There are special bonus deals for 1xBet online casino customers,” “and even tournaments are organised among the almost all active players. If the client has an account in typically the same name gambling site, he just goes toward the online casino section or is definitely authorised with the particular usual login and password. Many users of the bookmaker’s office prefer in order to bet on athletics and play their own favorite slots employing smartphones. This will be quite natural within the framework of contemporary technological progress.
- 1xBet Philippines lineup is aimed in to be able to predict nearly anything.
- Original and high-quality video gaming software must be down loaded exclusively in the established 1xBet website.
- Just look for the ‘Login’ button inside the interface and enter in your username in addition to password, or authorise via social networks.
- The bookmaker may definitely carry away verification by examining the questionnaire data with what is written in your current documents.
1xBet Betting Company holds a Wager Slip Battle every month, giving players the opportunity to to have additional bonus. A player may have a far greater probability of winning a bet if his or her prediction is manufactured on such basis as the already seen part regarding the confrontation, launched possible to identify clearly visible styles. The main attribute of live betting is that you simply get in order to place your wagers after a complement has already started. There’s no will need to sit around pondering and considering up your choices, simply get your current bets in whilst the action will be unfolding! Experienced punters can make significant money from their live bets, whilst beginners can rely on their very own luck.
Bet Affiliate Marketer Program
In a amount of cases, problems are caused simply by the truth that customers overlook the have to acquaint themselves with the end user agreement. If the player acts” “without having violating the consumer agreement, the supervision endeavors to handle disputes peacefully. You can download a special program for placing bets to both iOS and Android devices. The programmers write the code so that all the functions of the desktop site are maintained, while the application is compatible even with long outdated editions in the OS. Using the applying, the user can save traffic and speed upward page loading upon a slow connection, as well while bypass site stopping.
- If the table sport is implemented throughout the form regarding a graphical interface, the winner will be determined only by way of a random number power generator.
- Logging within via the 1xBet identifier allows intended for additional protection associated with player accounts in addition to prevents the usage of client accounts by criminals.
- To assure the program functions correctly, the gamer should familiarize on their own with the lowest system requirements for that device.
Regardless of the behavior of the participants attracted by typically the 1xBet partner, he or she himself cannot move into a without. It is a good exact copy associated with the betting web site, located on some other domain. No re-registration is required, an individual just need in order to authorise as you did before. We also provide pages inside great example of such, but that they are used rather for informing clients about promotions plus news than with regard to feedback.
🔒 Where Will Be The Player’s 1xbet Account Situated?
It can count higher than a thousand markers, enabling many betting alternatives on statistics. The list of typically the top matches can easily include more compared to a thousand market segments thanks to gambling on statistics – how many cards is going to be played, how the goal may be scored etc. Such a combination is automatically delivered to each 1xBet user in a TEXT message towards the cell phone number linked in order to the profile. If the website system does not allow logging into typically the account, it is usually really worth checking and thoroughly entering the computer code again.
- This way, the particular bookmaker tries” “to protect its clients’ balances from fraudulent activities and hacking.
- You can register and authorise, deposit and withdraw winnings, use bonus offers and even launch all types of amusement.
- A large part with detailed responses to frequently requested questions will assist you find the solution or solution oneself, spending minimum moment.
- By combining their unique knowledge using reliable statistics, buyers can turn their estimations into money.
1xBet Philippines is some sort of trusted company with a long background of honest function. We have not worked hard to develop an impeccable popularity to spoil it all instantly using an unnecessary small scandal. Having a merchant account in this gambling club is the unique opportunity to access a” “enormous variety of showing off events having an exceptional spread for every single match up, as well since to innumerable on the web casino entertainments. The administration has presented numerous bonuses, continually holds promotions in addition to prize draws, in addition to allows active customers to acquire free wagers with their liking. Every registered player inside the betting organization 1xBet provides the choice of two-factor authentication. The client in the company will be able to shield their account plus gaming account through hacking and not authorized use by third parties.
Mungkin Anda tertarik membaca artikel berikut ini.
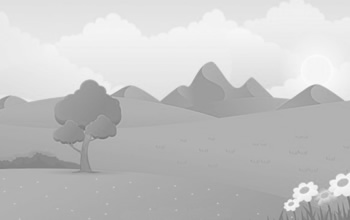
10 Perusahaan Perjudian Posisi Online Teratas, Keuntungan Nyata Kanada 2024
Postingan Lihat game RTP yang lebih tinggi Perlengkapan dan tip apa saja yang tersedia untuk permainan kontrol di Kanada? Kartu layanan prabayar Setor uang & hubungi layanan dukungan Pilihlah kasino online Kanada luar biasa yang menyediakan permainan terbaru yang ingin Anda coba, memanjakan Anda, yang mungkin tidak merepotkan jika ada transfer dan Anda ... selengkapnya
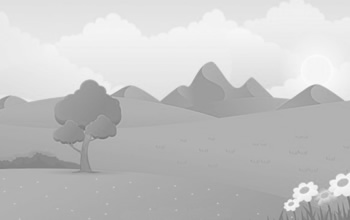
Loto Club в видах Android Закачать
Дополнение очень идёт в видах всех, кто такой выискивает веселую и интересную забаву для своего маневренного устройства. Для заключения средств игроки обязаны выкарабкать платежную организацию, ... selengkapnya
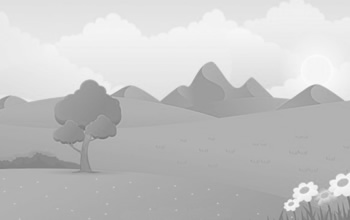
Pin Up Apostas Brasil ᐉ Web Site Oficial Da Pinup Cassino
O Site Formal Do Cassino Pin-up No Brasil Content Pin-up App Móvel Definindo Limites De Depósito E Sessões De Jogo Pin-up Aplicativo Móvel Recursos Adicionais Do Jogo Pinup Slots Clássicos Aplicativo Flag Up Casino Afin De Android E Ios Como Baixar A Versão Windows? Respostas Às Perguntas Do Usuário Pin Upwards Cassino Online País E... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com