- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Blogs
So, we only suggest gambling enterprises you to companion which have better app builders, guaranteeing you get a keen immersive gaming sense whenever. In the case of televised video game, players can often have fun with the smartphone otherwise tv remote control to put wagers instead of performing this thru a pc connected for the web sites. Typically the most popular alive specialist game offered at web based casinos is baccarat, blackjack, and you may roulette.
DraftKings – The benefit Gambling establishment
And, see reviews that are positive and you can views from other players and make certain the site uses SSL encryption to have investigation shelter. These incentives typically fits a percentage of your own initial put, giving you a lot more fund to try out having. For example, Las Atlantis Casino also provides an excellent $2,five hundred deposit suits and you will dos,500 Award Credit once betting $twenty five inside very first 1 week. A great provably reasonable game try a casino game you to definitely allows professionals be sure the outcomes to be sure it’lso are reasonable. It spends special technical to show that the outcomes is haphazard rather than controlled by the brand new gambling enterprise.
- The top online casino web sites offer many different game, generous bonuses, and you can secure systems.
- And make very first deposit during the a bona-fide currency internet casino is an exciting action that allows you to definitely start playing and you may potentially profitable large.
- The participants tell you their cards next last gaming bullet, plus the better give gains.
- Titles such as Cleopatra by IGT and you can Starburst because of the NetEnt are merely a few examples of your charming position game you to American participants love.
- Betway features a long-reputation record regarding the betting community and that is noted for its reliability and you may diverse gaming products.
- Because the business will continue to progress, participants is given numerous gambling games with modern jackpot game awards.
Finest Real money Online casinos inside the 2025
These types of video game are usually produced by top application team, making sure a top-high quality and you may ranged gaming feel. The new Come back to Pro (RTP) fee is a vital metric to own people aiming to maximize its earnings. RTP is short for the new part of all of the gambled money you to definitely a position or local casino games will pay returning to players throughout the years. Opting for games with a high RTP is significantly improve your chances of effective. If you are actually legitimate casinos might have particular negative ratings, the entire views will likely be generally positive. An excellent internet casino usually has a track record of fair gameplay, prompt winnings, and you will productive customer care.
These games function genuine investors and live-streamed http://www.grupafyi.pl/bez-kategorii/tien-thuong-song-bac-truc-tuyen-freeplay-tien-that-hoan-toan-mien-phi-choi-cung-cung-cap-cap-nhat-thang-1-nam-2025/ gameplay, getting an enthusiastic immersive sense. Finest gambling enterprises generally feature over 29 additional alive agent dining tables, ensuring numerous possibilities. Crazy Gambling enterprise provides typical promotions including chance-100 percent free bets to your live agent game. Ports LV Gambling enterprise application also offers free spins that have lowest betting requirements and some slot advertisements, making certain that dedicated players are continuously rewarded. Of a lot web based casinos offer self-different choices, delivering a supplementary coating out of manage to possess players who need to help you perform its betting models.
As well as, which have leading commission options such as PayPal, depositing and you may withdrawing is not safe and easier. When you enjoy from the one of the needed Us casinos, you’re also guaranteed to discover an authorized, top, and reliable program. The choices processes targets making certain you have access to safe withdrawals, many games of better organization, and lots of of the very attractive incentives on the market. Inside the membership procedure, profiles generally need provide a username, code, and personal information like their address, email address, and you will phone number.
Real cash Internet casino Ports Video game
To aid professionals navigate because of online gambling, we’ve collected a summary of more faq’s and you may offered concise responses. If you are enticing using their often big incentives and you may greater online game alternatives, overseas casinos have intrinsic threats. Such networks efforts outside of the legislation of us regulating government, making it challenging to address people issues or issues. Also, these sites may well not follow a comparable strict criteria from fairness and shelter one to regulated casinos on the internet perform. Yet, nonetheless they include enjoyable features for example added bonus video game and totally free spin cycles.
Slot Game
Such restrictions can include put limits, wager restrictions, and losses limits, making sure participants gamble within their form. Nuts Gambling establishment, El Royale Local casino, and you may SlotsandCasino is actually finest programs to have live agent games, for every giving various preferred titles and large-high quality streaming. If you would like black-jack, roulette, or baccarat, these casinos offer an engaging and you may realistic betting ecosystem. Ignition Casino, Cafe Gambling enterprise, and you can Bovada Gambling establishment are among the best online casinos in 2010, per giving book pros and you can a variety of game. Whether you want the brand new thrill out of progressive jackpots or even the proper issue away from vintage table game, these types of gambling enterprises have one thing to provide all types away from athlete.
Mungkin Anda tertarik membaca artikel berikut ini.
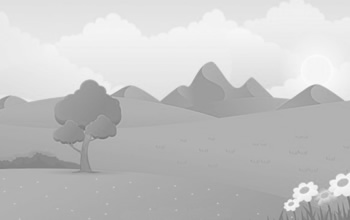
Скачайте Loto Club Диалоговый лотереи в видах loto club казино Android а также iPhone
Content Достижения игры в Loto Club выше аддендум | loto club казино Способы оплаты вдобавок заключения средств в LotoKZ Как скачать приложение Loto Club А как приняться играть получите и распишитесь деньги: фиксаци... selengkapnya
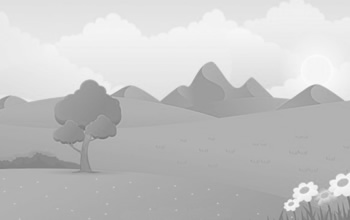
Top 10 On the internet Roulette Gambling enterprises 2024
Top 10 On the internet Roulette Gambling enterprises 2024 Posts Do i need to Enjoy Mobile Harbors To your Any Device? We Come across A new Casino Real time Casino games In the Cellular Casinos Should i Play Android Local casino Applications With A real income? 5 No deposit Bonuses Because of the Casinority The... selengkapnya
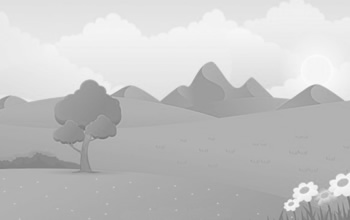
Скачать Мелбет получите и распишитесь Дроид безвозмездно, мобильное аддендум Melbet
Content Мелбет казино скачать – Употребления БК Мелбет Мелбет Скачать Бесплатно Похожие употребления MelBet (МелБет) – должностное адденда неповторимой из крупнейших букмекерских контор. Дает ... selengkapnya