- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Blogs
Governor Gretchen Whitmer closed away from for the Michigan Legitimate Gaming Operate (HB4311) for the December 11, followed closely by the fresh Legitimate Wagering Operate (HB4916) for the December 20, 2019. By 2017, the state had twelve online casinos in business – more somewhere else in america. Within the 2018, on the internet sports betting damaged the scene, due to regulations available with the fresh Jersey Local casino Handle Work and also the Nj-new jersey Office away from Gaming Enforcement.
Popular
Another treasure ‘s the DraftKings Rocket, DK’s creative spin to the popular freeze online game. Caesars Castle internet casino is belonging to Caesars Entertaining Amusement, Inc and you can are centered in 2009. The video game’s http://www.sucarpinteria.com/song-bac-truc-tuyen-tot-hon-tai-toa-an-hoa-ky-trang-web-duoc-mcw77-casino-quan-ly-nam-2025/ combination of means and you may possibility will make it popular certainly professionals. Guarantee the gambling enterprise uses highest-level encoding, ideally 256-portion, to guard your own and economic information. Discover customers reviews and analysis to gauge the newest casino’s reliability and avoid people malpractice or problems.
Electronic poker video game
Real time dealer online game provides revolutionized on-line casino gambling by providing a keen immersive and you can genuine sense. This type of online game feature actual-lifestyle buyers getting together with professionals within the actual-time thanks to a virtual platform, using the adventure away from a secure-dependent casino directly to the screen. Such networks offer a wide array of casino games, just like the ones that are inside conventional brick-and-mortar gambling enterprises, to your additional advantageous asset of to experience straight from the home. Engaging in online casino real money playing has become incredibly easier, achievable with just several clicks on your computer or mobile equipment. Particular casinos on the internet claim higher pay rates to have slots, and lots of publish payment percentage audits on their websites.
In control gambling is not just a good catchphrase you to definitely betting government spout in order to sound like they worry. Playing will be dangerous plus the growing emphasis on in charge betting strategies is intended to let people to deal with the gambling methods. Specific internet sites will offer a questionnaire that can be used to gauge if or not your or a family member features a gaming problem. Charge card gambling enterprises are usually a player favorite due to general convenience and convenience.
- Scratch cards, otherwise scratchers, is actually brief instantaneous-gamble game available at all of the greatest online casinos.
- The brand new country’s house-centered gambling enterprises as well as extended therefore the fresh legislation.
- That have a solid records on the betting globe, he brings in the-breadth analyses and reputable recommendations of several online casinos, enabling customers make informed choices.
- In the event you enjoy themed slots, online game including Esoteric Wolf and you can Wonderful Buffalo give an enthusiastic immersive and you will entertaining experience.
- The guy started while the a supplier in numerous online game, as well as black-jack, casino poker, and you may baccarat, fostering a feel one merely hands-for the experience offer.
El Royale Local casino provides alive broker online game running on Visionary iGaming, raising the reality of your local casino sense. Participants can also be interact with elite group and you may friendly traders, including a social element for the gameplay. The greater betting constraints in the live broker games from the El Royale Gambling enterprise offer a captivating issue for knowledgeable people. When deciding on a live gambling establishment, concentrate on the game options, respected application team, and you may betting restrictions that suit your look. Because of this, certain casinos on the internet today prioritize cellular compatibility. The newest mobile gambling enterprise application feel is essential, as it raises the betting feel to have mobile participants through providing optimized connects and you may seamless navigation.
This article will help you to browse the top casinos on the internet, emphasize popular online game, and show you the way to begin easily and you can properly. Alive specialist live online casino games host participants by effortlessly merging the new excitement from home-centered casinos on the morale from on the internet gambling. Such game ability actual people and you may live-streamed step, getting an enthusiastic immersive sense to have participants. The fresh judge design for United states online gambling is in a steady county out of flux. Alterations in regulations can affect the availability of online casinos and the security away from to try out during these networks. Opting for casinos one follow county regulations is paramount to guaranteeing a secure and you may equitable gaming experience.
While some says features legalized online gambling, anybody else have not, leaving participants in those claims with no possibilities but to turn in order to reputable offshore gambling on line sites. Once you play at best online casinos for real money, you can fund your bank account in a few means. Below are a few of the most well-known local casino percentage steps within the the usa today. We should say that deposit restrictions can differ away from local casino so you can gambling enterprise. Online slots games use up the largest amount from a gambling establishment’s online game choices. There are countless real cash slot online game at the best position sites on the web, as well as popular titles modified of house-founded servers.
More than just a-game out of opportunity, on-line poker pits you from almost every other players inside a combat of experience and means. The fresh digital realm provides preferred casino poker variations such Mississippi Stud, 3-card casino poker, and you can live dealer Hold ’em for the forefront. The fresh betting diversity becomes a pivotal grounds here; whether you are a casual athlete otherwise a high roller, the best casino is always to match your financial allowance.
✅ Reputable customer service
Created in 2002, Sunlight Palace Casino are an experienced on the on the internet betting world. It’s participants a trusted system run on Real time Gaming (RTG). Of a lot web based casinos in the us deliver the solution to play trial versions of the casino games. These types of demo versions provide the exact same gameplay sense since the actual currency online game, allowing you to gain benefit from the adventure and you can activity rather than betting one currency.
Gamble $step one, Score $one hundred within the Casino Incentive
A softer and you may safe deposit procedure comes to verifying the brand new deposit possibilities, guaranteeing exchange times is actually prompt and you will legitimate, and you can examining the lowest and you can limit put restrictions. Real time roulette, another well-known option, includes Eu and you may American alternatives. Unique offerings such Local casino Floor Roulette and Live Vehicle Roulette increase the variety and you can excitement of one’s game. The typical minimum wager to have alive roulette is actually $1, therefore it is open to an array of participants.
Mungkin Anda tertarik membaca artikel berikut ini.
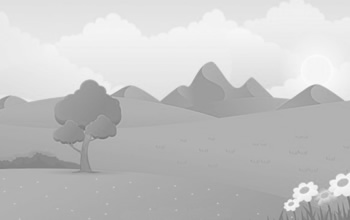
10 Best Real cash Online slots games Sites of 2024
Of several web based casinos now offer cellular-amicable systems otherwise devoted apps that enable you to take pleasure in your favorite position game everywhere, each time. On this page, you’ll see intricate reviews and you may suggestions round the certain kinds, ensuring you have everything you need to create advised conclusion. Whether your’re looki... selengkapnya
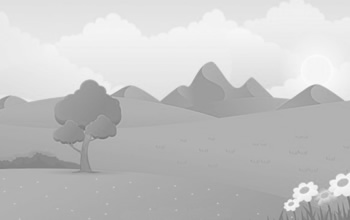
Finest 2024 No-deposit Added bonus Casinos in the Us Allege Free Currency
Articles The newest Godfather Position Superb Slots To play To your GGBET Gambling enterprise Free Spins for the Popular Harbors Joining a free account No-deposit free potato chips is a kind of added bonus offered by web based casinos that allow participants to play table game instead of being forced to invest their particular money... selengkapnya
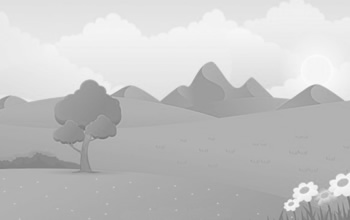
Bahisçi ve Kumarhane Pinco Girişi Resmi Web Günlüğü Pinco Casino Rusya'yı alın
İçerik Pinco Bet'te spor bahisleri: Oyunculara indirimler sunan yeni bahis sitesi Pinco Pinko kumarhanesi – Rusya'da resmi dergi ve onay Ücretsiz bahisler Bir video oyunu agio-conto'yu hacklemek için, on sekiz yaşını tekrarlamak, bahis koşullarına ve ayrıca bahisçinin emirlerine rıza ile yanıt vermek kalır. "Favoriler&quo... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com