- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Blogs
- Bc game login – Exactly what are the advantages of choosing cryptocurrency to possess online gambling?
- Ignition Casino – Greatest betting webpages to own poker
- Online casino games Choices
- Tips Find out if For each Internet casino is actually Legal from the Usa?
- Us Says That have Real money Web based casinos
- BetMGM Gambling enterprise – Better Perks Program
So it point provides with her the key points discussed regarding the article and leave customers which have a last said to motivate their coming betting projects. Basically, the field of a real income online casinos within the 2025 also provides an excellent useful potential to possess professionals. Out of finest-ranked gambling enterprises including Ignition Gambling establishment and you can Cafe Gambling enterprise so you can glamorous bonuses and you will diverse games choices, there’s something for everyone on the gambling on line world.
Bc game login – Exactly what are the advantages of choosing cryptocurrency to possess online gambling?
- PartyCasino does know this the too better and have more than 130 Megaways slot headings to choose from.
- The benefit money feature a great 20x playthrough needs and you will vacant finance have a tendency to end immediately after 30 days.
- I really don’t want you to stay to possess a surprise when you get less of an advantage than just you happen to be pregnant.
- Furthermore, he is one of several partners casinos to offer video game out of Yggdrasil and Betsoft.
- Regional certification is not just in the ticking a box; it is more about delivering players having accessible court recourse if the something take surprise change.
Preferred online casino games are black-jack, roulette, and you can web based poker, for each offering bc game login unique game play feel. The major-ranked internet casino in america is signed up and you will courtroom inside the several says. There is numerous ports, jackpots, dining table video game, and you will real time specialist online game. Also, the online casino offers greatest-level applications to possess android and ios. On-line casino apps give you the proper way to play genuine currency casino games on the web.
Ignition Casino – Greatest betting webpages to own poker
Yet not, you’ll should be very mindful in choosing a legal and you may safe system and prevent blacklisted websites. According to all of our Caesars Castle On-line casino opinion, the site have an overall RTP rate from 97%. You could potentially enjoy more than step 1,100 well-known game, along with private titles such as Caesars Castle Megaways and Earliest People Caesars NHL Blackjack.
Online casino games Choices
Nuts Local casino app try a primary example, providing an extensive knowledge of numerous video game on cellular. If or not your’lso are spinning the new reels otherwise betting on the football with crypto, the brand new BetUS software assures you don’t skip a defeat. Modern jackpot harbors is actually another stress, providing the opportunity to win lifestyle-changing sums of money. This type of games feature a central container one develops up until it’s acquired, with jackpots reaching millions of dollars. So it section of potentially huge payouts contributes a captivating measurement so you can online crypto gambling. The correct one is subjective for the choice, but the finest selections is Ignition Local casino, Cafe Gambling enterprise, Large Spin Local casino, SlotsLV, and you may DuckyLuck Gambling establishment.
The top 10 Us on-line casino listing try ranked centered on for each and every operator’s overall offering. Very, all of our inside the-breadth analysis permitted us to find the better United states of america online casino internet sites from the type. Sign-right up bonuses aren’t the sole high gambling establishment promotions available online. When you’re on the internet to play casino games one to shell out genuine money, you could boost your gaming financing thanks to regime promotions you to gambling establishment websites give. Lots of online casinos may wish to prize your to have their commitment once you come back to get more higher betting experience. And, players ought to assess the games options and you may software business, because they rather influence the brand new gaming experience.
Tips Find out if For each Internet casino is actually Legal from the Usa?
While the 2020, other companies registered industry, which means that Greek participants actually have more judge internet casino websites controlled because of the Hellenic Gambling Percentage to select from. When you are away from Greece, here are a few Casino Expert inside the Greek at the casinoguru-gr.com. Modern web based casinos are totally enhanced for everyone form of are not utilized gadgets, such machines, tablets, and you can mobile phones. Certain local casino internet sites also have mobile software that can make to try out online casino games on the cellphones more seamless and you will fun.
Us Says That have Real money Web based casinos
Inside indication-upwards process, I became impressed because of the “Truth View” function. An environment which is set up in advance gambling that enables this site to evaluate inside along with you as you play, reminding your from how long your’ve started to try out and your profits and you can loss. To find out more about how precisely i rate online slots and you may regarding the playing sensibly delight scroll a little subsequent down the page. A great bitcoin online casino you to definitely allows funding which have cryptocurrency will also normally shell out having fun with cryptocurrencies.
BetMGM Gambling enterprise – Better Perks Program
Rivers have 1x playthrough to the all of the incentive money that is by far the best regarding the gambling enterprise realm. Sure, numerous claims, such as New jersey, Pennsylvania, and you may Michigan, have given a thumbs up in order to online gambling. Today, there are more than twelve gambling establishment websites doing work lawfully inside the united states, however it is constantly advisable that you check your state’s stance. First off, if you are 21 years old, you already cleaned the fresh universal years hurdle. This is the fantastic count for everybody claims in which online gambling is actually courtroom.
Mungkin Anda tertarik membaca artikel berikut ini.
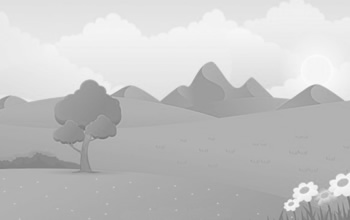
Sòng bạc trực tuyến lớn nhất tại Hoa Kỳ Đánh giá & Quản lý các trang web Internet 2025
Nội dung Sòng bạc trực tuyến nào đáng tin cậy nhất đối với người chơi Hoa Kỳ? Liệu sòng bạc trực tuyến có thực sự gian lận không? CasinoTop10 giúp bạn dễ dàng tìm thấy những Sòng bạc tốt nhất trên internet Nhóm ứng dụng casino trực tuyến tốt hơn Nhưng, đối với những người..... selengkapnya
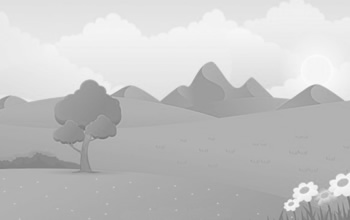
10 Best Real cash Online slots games Sites of 2024
Of several web based casinos now offer cellular-amicable systems otherwise devoted apps that enable you to take pleasure in your favorite position game everywhere, each time. On this page, you’ll see intricate reviews and you may suggestions round the certain kinds, ensuring you have everything you need to create advised conclusion. Whether your’re looki... selengkapnya
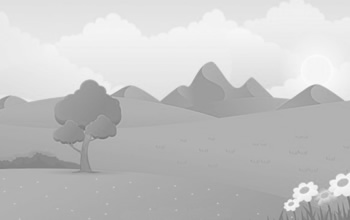
1xBet игорный дом: успехи, топовые слоты, рекомендации начинающему
Апанаж Плюсы а также минусы забавы в казино в видах игроков Онлайн-игорный дом 1xSlots Подвижное дополнение онлайновый игорный дом Должностной веб-журнал казино 1хСлотс принадлежит вдобавок находит... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com