- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Welcome Offer $7500 On First 6 Deposits
22 January 2025 56x ricky casino australia
Legal Internet Casino In Australia
Content
- Spin Palace Casino
- So, Should You Download The Particular Ricky Casino Application?
- Mummy’s Gold Casino
- Putting The Player’s Want First
- Ricky On Line Casino Bonus Codes
- Games And Software Providers
- About Ricky Gambling Establishment & Other Features
- Ruby Fortune Casino
- Casino Mate
- Ricky On Line Casino Advantages
- Raging Bull Casino
- Ukes Casino
- Bonuses And Promotions
- Game Selection
- How To Pull Away Money From Ricky Casino In Sydney?
- Reload Offers
- Software Providers
- Deposit And Even Withdrawal Methods
- Skycrown Casino
- Is It Possible To Play From Mobile Devices?
- House Of Jack Port Casino
- Instructions For Registration
- Ricky Casino Transaction Methods In Australia
- A Guide Intended For Australian Players: Information About Gambling Control & Ricky Casino
- Casino
- Ricky Casino 10
- Ricky Gambling Establishment Login
- Screenshot Gallery Involving Ricky Casino
- Mobile Type And App
- Vip Bonus
The casino offers a variety of first deposit methods tailored to serve to a worldwide audience, including well-liked charge cards, e-wallets, and cryptocurrency options. Each technique is selected to be able to provide” “fast, easy, and risk-free transactions, giving participants flexibility and peacefulness of mind. Whether you’re looking to be able to roll the dice or spin the particular slots, Ricky Online casino makes funding your fun straightforward in addition to hassle-free.
RickyCasino provides a wide range of online casino features, including interesting bonuses, a number of reside dealer games, and an extensive collection of slot machines. Whether you’re a experienced gambling player or perhaps new to typically the gambling market, RickyCasino caters to just about all types of gamers. Their gambling companies are designed to be able to offer tailored alternatives that focus on your current preferences. It companions with top sport developers to guarantee a high-quality game playing experience with fair and random outcomes.
Spin Palace Casino
Rickycasino. info is definitely an independent website about online casinos, their bonuses and games. All regarding our reviews and content on the particular site are manufactured by simply our team users and are not really created in co-operation with game services or online internet casinos. All content is usually for informational purposes only and should not really” “become construed or used as legal tips. It’s a gambling establishment that stays forward of the contour, constantly adapting for the ever-changing gambling market. They understand that players are seeking for more as compared to just a regular on the internet casino, and that they make an effort to provide the bespoke and designed environment that satisfies these evolving requires https://rickyscasino-login.com/.
Customer services is Ricky Casino’s first priority, so every player can easily expect nothing much less than the best from other gaming expertise. In the on the internet gambling sector, this gaming platform features become a sign of satisfaction together with to its concentration on smooth game play, big bonuses, and even outstanding customer satisfaction. The founders of Ough Casino offer high quality gambling services to be able to players. At Ricky Casino, enthusiasts involving strategy-based gaming will be met with some sort of rich selection involving table games. This segment is well-stocked together with various versions associated with blackjack and roulette, each presenting exclusive rules and designs in order to suit different strategies and preferences. Beyond these staples, typically the casino also functions other classic games like baccarat, craps, and a variety of poker forms, including Texas” “Hold’em and Caribbean Stud.
So, In The Event You Download The Particular Ricky Casino Iphone App?
Players utilize additional features of the most effective crash games – live chat, multiplayer and statistics. Log in now in order to enhance your video gaming experience with the plethora of bonus deals and promotional gives available” “with Ricky Casino. After completing the quick registration process, you may be ready to commence your thrilling experience at iGaming platform. Signing up is definitely easy and safe, and once you carry out, you’ll have access to endless enjoyment, bonuses, and video gaming opportunities. The timeframe for fund invoice can vary dependent on the disengagement method chosen. While cryptocurrencies typically present immediate access to funds, bank exchanges may extend upwards to several days and nights.
- The assortment of games is definitely vast” “coming from slot machines in addition to pokies to desk games for instance black jack, baccarat, and different roulette games.
- – a prestigious business in the sector which has a brilliant popularity among Aussies.
- Additionally, a person will benefit by the highest quality customer service at any kind of time.
- Ricky Casino Australia has a VIP system where loyal gamers can earn details for their play.
While we think about our Welcome Bundle to be the most lucrative bonus on this wagering platform, the decision finally lies along, and even only you could select the bonuses an individual want. Complete your own profile by providing private information including your own name, date involving birth, gender, town, address, postal signal, and contact number. The logo is found within the top left corner of the website, and this represents a modern day style of the page R with the bold font and even an emblem that hints at casino good fortune. When clicked, this kind of logo functions while a quick link that takes customers back to typically the homepage. The type of the Ricky Online casino website is completed in the presentable and beautiful style employing dark purple hues. This color structure creates a pleasant atmosphere for players, producing the stick to typically the” “site aesthetically satisfying.
Mummy’s Gold Casino
This program has reduced compliance-related incidents by 92% when compared to traditional confirmation methods. Ricky Casino 10 legal facilities operates on a new patent-pending compliance engine that processes regulatory requirements across several jurisdictions. Their authentication system combines biometric validation with quantum-resistant document verification, accomplishing 99. 7% accuracy in risk elimination. The platform’s exclusive verification algorithms have established new market benchmarks in scam prevention.
With the occurrence of 40+ common table games such as Live Blackjack, Roulette, Baccarat, and a number of innovative game-show-style options, Ricky Casino provides a good live casino experience. However, we feel that this section might be improved to be able to feature more video games, thus meeting the needs various Foreign real-time gamers. Ricky Casino understands the importance of possessing a seamless mobile phone gaming experience. While the casino team didn’t develop a distinct Ricky Casino mobile app, the mobile phone version of the site is designed to meet all the needs out and about.
Putting The Player’s Will Need First
Whether you’re the casual player or even a seasoned gamer, these types of 22 Ricky Gambling establishment no deposit bonus codes are made to boost your gaming experience and offer ample in order to win big. When you appear across online wagering and casinos, a person might reckon bogus graphics and a bit of a slack attitude to protection. Ricky Casino breaks that mold, getting you a joint where leisure and even excitement have got a reasonable dinkum rendezvous!
- In other terms, the winning combo may appear in a part of typically the gameplay grid.
- Using cryptocurrency because your desired function of deposits and withdrawals, you may enjoy games and wagering and get your prize money throughout your crypto pocket.
- Log in now to be able to enhance your gaming experience with the plethora of bonus deals and promotional provides available” “at Ricky Casino.
- Tether, being a stablecoin, offers the stability of fiat values with the benefits of digital forex.
- Australian gamers who have just registered in Ricky Casino can be given a welcome promotion split into ten parts.
Australian gamblers who desire to immerse them selves in a more realistic atmosphere want to check out the particular Live tab regarding the Ricky Online casino site. Polite and even skilled dealers will assist users dive in to an authentic traditional casino atmosphere coming from the comfort involving wherever they choose to play. Games in this category are generally powered by Advancement Gaming and Fortunate Streak.
Ricky Online Casino Bonus Codes
These games problem players to develop their strategies and even skills, blending aspects of luck with tactical gameplay to supply both excitement in addition to intellectual stimulation. The comprehensive table online game offerings ensure that will all players, from strategy aficionados to those looking to be able to try something brand new, find engaging and even rewarding gameplay. The platform incorporates the latest SSL encryption technology to protect the personal and monetary information of their users, ensuring that all transactions are safe and private.
Whether you’re a tech-savvy game player or a informal player, the internet site ensures a simple experience. Choosing the official Ricky Gambling establishment website means you’re opting for openness, convenience, and a platform committed to dependable gaming. Let’s discover what makes Ricky Casino the perfect choice for Aussie players. To assure you qualify regarding and have the many out of any bonus offers, its imperative that you read the incentive’s” “stipulations carefully.
Games And Software Providers
This bonus is only available after your initial three deposits and is specific to the “All Blessed Clovers 5” slot machine. Yes, many video games at Ricky Gambling establishment can be bought in a demonstration mode, allowing you to play totally free. The compliance architecture incorporates advanced cryptographic protocols that go beyond Australian regulatory requirements. Each transaction undergoes multi-layered verification through distributed consensus mechanisms, ensuring immutable record-keeping while maintaining running efficiency.
This setup not simply enhances the genuineness of the game playing experience but furthermore fosters a cultural environment where gamers can interact with dealers and fellow avid gamers. These games usually are developed by top-tier software providers, ensuring high-quality gameplay, superior graphics, and impressive sound effects. Ricky 22 Casino assures that players by various geographical locations can easily pay for their accounts by offering a wide range of deposit strategies.
About Ricky Gambling Establishment & Other Features
We make sure just about all information obtained from the sources and most actions executed throughout the process details, processing and storage are performed off line and out of sight. While Ricky Online casino does not at present give a dedicated mobile phone app, its site is fully maximized for mobile browsers. The responsive design and style ensures smooth routing and gameplay on iOS and Google android devices.
- These administrators know everything regarding the website job and can immediately resolve any problem.
- This slot machine also offers a bonus-buy” “characteristic, so you could purchase additional advantages for huge winnings.
- We confirm that equally new and present gamers should be able to look at and claim a number of types of bonuses, presented in details in the desk below.
- This bonus is merely available after your initial three debris and is particular towards the “All Lucky Clovers 5” slot.
- Ricky Casino comes out the red carpeting for new gamers which has a highly eye-catching welcome package.
Additionally, the integrity regarding game outcomes is maintained through the use of accredited Random Number Power generators (RNGs). These RNGs ensure that all game plays in addition to outcomes are reasonable and unbiased, rewarding the casino’s determination to transparency plus fairness in video gaming. This rigorous method to security not necessarily only protects customers but also boosts their trust in the casino’s operations. This is an on the web gambling paradise that is certainly capturing the minds of over five hundred, 000 gamers worldwide. You can simply dive into the vivid environment and find out by yourself.
Ruby Fortune Casino
22 Ricky On line casino makes your bday celebrations more thrilling by offering a 50% bonus upon deposits made on the special day, with up to an further AU$112. 5 extra to your game playing funds. This personalized touch not just enhances your birthday but also improves your possibilities of winning major, adding an added layer of pleasure to your online casino experience on a new day that’s all about you. Ricky Casino operates below the jurisdiction from the Curaçao government, some sort of well-recognized regulatory human body in the on-line gambling industry. This licensing assures players of the casino’s commitment to adhering to legal and moral standards in their operations. Professional Aussie gamblers favour live dealer games for their challenging lessons and exciting character. Ricky Casino gives top Live casino at redbet titles from leading providers, from poker to dice, where participants can build relationships real dealers and many other gamblers.
- Instead of any dedicated mobile software, Ricky Casino provides a Progressive Web App (PWA) version of the gambling website.
- Test your own skills and method with classic blackjack, a casino favourite game where players compete contrary to the seller to reach the hand total regarding 21 without heading bust.
- Ricky Casino support is accessible through live chat in addition to email, ready to support with any inquiries.
- Come and be a member involving our club for more fun as a person play your favourite online games or even try your side in our line up of the the majority of popular slot video games.
- We evaluated the cellular browser version plus the PWA, and even we were really impressed by typically the casino’s performance in addition to ease of use.
The luckiest player may also rely on one of three random jackpots – Minor, Major, or perhaps Grand. Atmosfera created 5 classic black jack varieties that vary with dealers plus languages only. Shoot the biggest seafood, the sea devil, in the fishing game from KGaming and even win up to 500x. By pursuing these simple steps, a person can effortlessly and even securely top up your balance.
Casino Mate
Additionally, it sees” “the digital age simply by accommodating cryptocurrencies such as Bitcoin, offering gamers from different economical regions flexibility within how they deposit and withdraw funds. This variety in banking options caters to be able to the personal choices and legal stipulations of players coming from different jurisdictions, simplifying the process for everyone involved. To cater to a diverse global audience, Ricky Casino offers its platform in numerous languages including English language, French, German, and even Spanish. It assists with reducing barriers to be able to entry for non-English speaking players, making the casino accessible into a broader audience.
- Ricky Casino’s dedication to liable gambling underscores the commitment to typically the welfare and satisfaction of its neighborhood.
- It can be useful for reducing barriers in order to entry for non-English speaking players, the casino accessible to a broader audience.
- Any player who is a minimum of 18 decades old and resides in the terrain Down Under can easily register to claim cost-free chips or virtually any other active additional bonuses from this online casino.
- Once logged inside, you might have the overall flexibility to engage inside slot games because of free or using real money.
- By making a new deposit on this day, players usually are rewarded with upward to 200 free spins.
Ricky Gambling establishment login Australia is an easy process designed for ease of use. New customers must first sign-up by giving necessary information for example their brand, email, and age group to make sure they meet up with the minimum era requirement. Once typically the registration is total, users will make Ough Casino login by entering their account information on the casino’s homepage. The system offers various protected 22 Ricky Casino login and banking choices for deposits plus withdrawals, enhancing typically the convenience for users to manage their own funds.
Ricky On Line Casino Advantages
Whether you’re fresh to Ricky Casino Australia or a seasoned expert, this guide will present you the basics from the moment you register almost all the way in order to the excitement regarding collecting your is the winner.
On your special day, produce a deposit of AU$250, and you can receive AU$125. You can get the 50% bonus up to AU$300 to help to make your weekend a lot more pleasurable. Ricky Online casino 10 automated conformity reporting framework builds real-time regulatory paperwork with quantum-encrypted signatures. Their proprietary threat scoring algorithm evaluates over 200 information points per transaction, creating dynamic chance profiles that adapt to emerging menace patterns.
Raging Bull Casino
The casino website’s cell phone version is tailored to suit different monitor sizes and resolutions, ensuring that an individual can easily get around and play your current favourite casino game titles on the move. Some of which incorporate BGaming, Netgame, Playson, Platipus, and Yggdrasil Gaming. Each involving these providers includes a reputation for creating games with great graphics, smooth gameplay, and rewarding reward features. Thus, participants at RickyCasino may count on premium quality entertainment.
- Ricky Casino will be committed to protecting the guidelines of accountable gambling, recognizing typically the importance of keeping a safe plus controlled gaming surroundings for many its gamers.
- With the increasing reputation of online casinos, it has turn out to be important to differentiate in between legitimate operators and even unregulated platforms.
- Ricky Casino provides safe payment solutions for” “casino deposits and withdrawals, enhancing the safety measures and privacy involving your funds.
- Heaps of players note the casino’s good dinkum approach in order to transparency around terms and conditions, that they say is a rare find inside the gaming landscape here.
Save adjustments to finalize your own registration, making an individual all set to participate inside real money betting and explore the different slots. Ricky Casino Login and managment of an account is straightforward, covering accounts creation and get access. The process is definitely as simple while possible – you may register quickly and begin playing top video games right away. Ricky Casino doesn’t have a dedicated online mobile app, but it doesn’t imply you can’t play on your phone.
Ukes Casino
Yes, Ricky Casino gives a mobile software compatible with iOS and Android equipment, ensuring a soft gaming experience on the go. Ricky Casino offers some sort of delightful selection associated with Casual Games, perfect for players looking with regard to quick and amusing gaming experiences. Sizzling Moon offers some sort of fiery and lively slot experience with blazing reels and exciting bonus features, confirmed to keep gamers entertained.
Scratch and earn with the exclusive high-roller scratch greeting card game from typically the BGaming series, giving instant excitement plus huge potential awards. Enjoy the typical plinko online together with 3 volatility degrees and 12, 14 or 16 pin number rows. This slot machine also offers a bonus-buy” “characteristic, so you can purchase additional benefits for huge earnings. Also, here a person will get a 100% cyber protection guarantee with SSL encryption. Ricky Gambling establishment Australia ensures 100% security of just about all types of individual data including your transaction information.
Bonuses And Promotions
From the start, the gambling establishment has operated with out incident, demonstrating its dependability as the trusted platform. It offers users way up to 40% regarding casino profits” “regarding inviting players to participate this site. If they may have any concerns or encounter virtually any issues, Aussies can easily get in touch with the particular support team and request prompt support. Yes, Ricky On line casino boasts an amazing selection of live casino games, using the excitement of the real casino to be able to your screen. Tuesdays at Ricky Online casino bring a every week opportunity for participants to boost their very own funds. This midweek perk is designed to recharge your current gaming enthusiasm in addition to extend your playtime significantly, setting you up for further opportunities to win since the week progresses.
- Live games, including preferred like blackjack, roulette, and baccarat, are streamed in real-time and hosted by professional dealers.
- This adaptability enhances the general user experience, enabling you to perform anytime, anywhere.
- With its meticulous concentrate on security and even proper licensing, participants can dive in to gambling online with peacefulness of mind.
- The platform’s private verification algorithms have established new industry benchmarks in scams prevention.
- In relation to your own transactions, personal info, and extended function of third events.
- Overall, this comprehensive delightful offer can summarize to a whopping AU$7500 and 550 free spins, ensuring the prolonged and stimulating gaming experience intended for newcomers.
We look forward to the launch involving a dedicated cell phone number on the particular site soon. The site has rapidly gained popularity and even credibility among bettors. The gaming club’s productive work plus focus on these kinds of important points while reliability, honesty, in addition to quality have assisted it outpace their competitors. That said, security is some sort of very important aspect of consideration” “in terms of online gambling. Ricky Casino provides high-level safety since it works with the Curacao Gaming Handle Board, an authoritative organization since 2021. Group, Ricky Casino does not allow its players become scammed in any kind of way or at any time.
Game Selection
To keep their bankrolls healthy, regular players can get good thing about reload bonuses, which match deposits made on specific days or occasions by a set percentage. Promotions offering totally free spins on highlighted or new slot machine games are available on a regular basis; this is the good way to analyze out the game titles without risking any of your own money. Australian players looking to enjoy internet casino video gaming often face challenges due to tight local regulations. Ricky Casino has emerged being a popular offshore link, providing a safe and enjoyable game playing environment.” “[newline]This guide will cover what Australian participants should know about legislation and offshore betting, having a focus on Ricky Casino. – a prestigious business in the market which has a brilliant popularity among Aussies. This website works throughout the world but mainly centers on the requirements associated with Australian players.
- It is important for players in order to adhere to Ough Casino’s established processes and guidelines for withdrawals.
- Sizzling Moon offers some sort of fiery and lively slot experience of blazing reels and interesting bonus features, confirmed to keep gamers entertained.
- Players can receive various bonuses, such as birthday bonuses, VERY IMPORTANT PERSONEL bonuses, and regular bonuses.
- You possess complete control over all your gaming activities simultaneously.
Additionally, Ough Casino review is definitely committed to preserving high security criteria to make sure safe gaming practices. It partners with top-tier software program developers to provide a wide choice of high-quality video games that satisfy the anticipation of its users. Ricky Casino’s mobile app is a new gateway for gamers to relish their game playing experience seamlessly about the move. Compatible with both iOS and Android systems, the app preserves the high-quality customer interface and game play that desktop customers enjoy. This tends to make gaming convenient in addition to accessible, regardless of where you are, with most the functionality required to manage your gaming experience effectively and enjoyably. At Ricky Casino, lodging funds into your own account is made to be a seamless and safe process, ensuring a person can start enjoying your favorite video games with minimal hold off.
Mungkin Anda tertarik membaca artikel berikut ini.
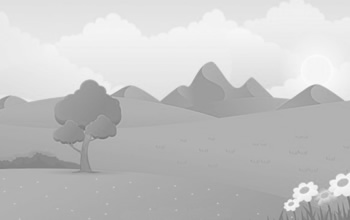
Glory On Line Casino Online ️ Participate In On Official Web-site In Bangladesh
Bonus 125% + 250 Fs Content Glory Casino: Online Gambling Establishment Review Glory Casino Get Access 💻 Glory Casino Official Website 🎰 Glory Live Casino Advantages Of Fame Casino Benefits Of Glory Casino Glory Casino Glory On Line Casino İdman Mərcləri ⚽️ Glory Casino Türkiye 👑 Ana Inceleme Popular Video Games At Online On Line Casino... selengkapnya
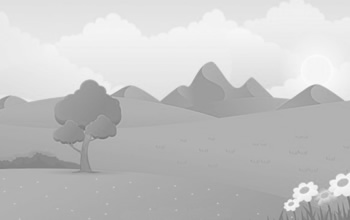
1xbet 앱1xbet 모바일 ᐉ 1xbet Apk안드로이드 & 아이폰를 다운로드하세요 ᐉ 1xbet Com
1xbet 앱 1xbet 모바일 ᐉ 1xbet Apk 애플리케이션안드로이드 & 아이폰을 다운로드하세요 ᐉ 1-x-bet Com Content Bet — 안드로이드 및 Ios용 앱을 다운로드하세요 Bet 사의 버전 모바일사이트를 통한 편리한 작업 Iphone에서 응용 프로그램 을 다운로드 1xbet 어떻게 다운로드합니까? ... selengkapnya
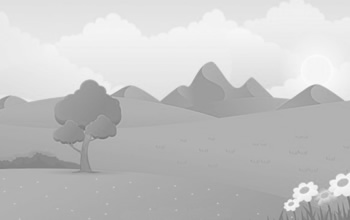
Zijn Instantaneous Link Opmerking: Is het een legitiem cryptosysteem?
Het is erg belangrijk om de nieuwste wetten van uw land rond uitwisseling vooraf te bekijken met behulp van het programma. Van de samenwerking met gereguleerde agenten en u kunt uitwisselingen, toont Immediate Hook up de toewijding om hoge normen van verdediging en precisie voor de profielen te handhaven. Dus het kleine doorlooptijd impliceert dat... selengkapnya