- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Content
Cryptocurrencies is revolutionizing the way in which players transact that have online casinos, offering privacy, protection, and you may price unmatched from the old-fashioned financial tips https://bhawananutrifit.com/2025/02/06/%e0%b9%80%e0%b8%81%e0%b8%a1%e0%b8%84%e0%b8%b2%e0%b8%aa%e0%b8%b4%e0%b9%82%e0%b8%99%e0%b8%ad%e0%b8%ad%e0%b8%99%e0%b9%84%e0%b8%a5%e0%b8%99%e0%b9%8c%e0%b8%97%e0%b8%b5%e0%b9%88%e0%b8%a2%e0%b8%b4%e0%b9%88/ . Bitcoin and other electronic currencies facilitate close-quick dumps and you may distributions while maintaining a premier amount of privacy. Taking into consideration the above mentioned, you should be careful of web based casinos acknowledging You players of much more claims than the six secure here. Sweepstakes gambling enterprises the next and the United states web based casinos on this page make up their pond from legal gambling alternatives.
It’s chill that they allow you to sift through online game because of the supplier — not a thing the thing is every-where. You can receive their points during the MGM physical towns along side nation. Instead, you might replace him or her for on the internet bonus loans to electricity your own on the internet gambling training. Caesars Castle on-line casino is actually belonging to Caesars Interactive Entertainment, Inc and is actually centered in ’09.
Reputable Software Company
Bally, a legendary name on the gambling world, has recently lengthened the extent by the unveiling online casinos inside the The brand new Jersey and you will Pennsylvania. The newest professionals in the Borgata is invited which have a great $20 no-put extra right off the bat. And in case you happen to be prepared to put, you will find a good 100% match incentive waiting for you, up to $step one,000. The competition among gambling brands in the more successful segments is actually tough. The fight ranging from You.S. operators isn’t just inner — they’lso are up against overseas web sites promising the newest moonlight plus the celebrities. For anyone merely dipping their toes within the, picking the best program and guaranteeing it’s safer if you are trying to find more nice incentives might be daunting.
Top ten Real money Web based casinos to possess 2025
Step one is always to look at the casino’s official website and find the fresh registration otherwise indication-upwards key, always prominently displayed to your website. Mega Joker from the NetEnt shines since the highest payout position games currently available, offering an extraordinary RTP of 99%. That it vintage position games now offers an easy yet rewarding experience to own people that find higher production. Another high RTP position games of NetEnt are Blood Suckers, presenting a vintage horror theme and an RTP from 98%.
- When the movies harbors are more your look, you’ll see added bonus-manufactured game that have five reels.
- E-take a look at seller VIP Popular is recognized at the best on-line casino web sites.
- Of greeting bonuses in order to 100 percent free added bonus rounds, an educated United states of america web based casinos in the us offer a range from offers to improve the gaming feel.
- The advantage dimensions, in the limitation $250, might not be as the popular with big spenders because perform getting in order to a casual athlete.
To aid players browse due to online gambling, we’ve gathered a listing of more faq’s and you will given to the level answers. When deciding on a bona fide currency online casino, it’s necessary to be mindful. Focus on the secret issues, such as legitimate permits and you can self-confident user feedback. As well as, keep clear away from red flags such as unresolved problems and you may unclear licensing suggestions. That way, professionals is also go on its playing experience in trust and you will security.
Signed up local casino internet sites need invite separate audits of its online game and payouts. Thus, signed up sites is the trusted and most dependable online casino web sites in the usa, including gambling sites you to undertake Skrill. The brand new dining table game options and impresses, that have antique games and you will innovative versions, for example Room Intruders Roulette. It also features a private BetRivers real time broker blackjack video game you will not discover any kind of time other online casino. You can discover an excellent welcome extra utilizing the BetRivers Michigan added bonus code. Find complete details within our BetRivers MI local casino opinion, or proceed with the secure hook lower than first off to play.
A real income Online casino games with a high Profits
Periodically, the fresh also provides lower than might not match the gambling enterprises i emphasize. This happens if the provide is not currently available on your own part. In this case, we will direct you next better give available to choose from.
If the a password is needed, we are going to render it otherwise direct players to help you in which they’re able to see it. Yet not, as with any kinds of betting, it involves exposure, and there’s no make sure of cash. The brand new gambling enterprise also offers 600+ online flash games, most of which are preferred Practical Gamble headings along with the rest coming from the likes out of Hacksaw, BGaming, OneTouch, and Spin Betting. Games by the Finest Real time and Risk Alive fill out the brand new gambling establishment’s live agent lobby, in addition to all the classics such blackjack, roulette, baccarat, and you can sic bo. There are also around 20 exclusive game labeled ‘Stake Originals’.
Created in 2002, Sun Palace Gambling enterprise try an experienced on the on the web betting community. It’s professionals a reliable system powered by Realtime Playing (RTG). Recognized for their long-condition profile and you may diverse online game possibilities, Sunshine Palace will bring a strong on-line casino sense, that includes big incentives and a range of financial possibilities. There are numerous better online casinos available, but that doesn’t mean it work in all of the court condition otherwise have the online game choices you are looking for.
Mungkin Anda tertarik membaca artikel berikut ini.
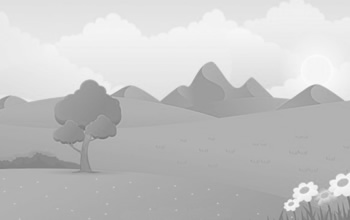
Закачать Мелбет получите и распишитесь Дроид бесплатно: адденда Melbet и скидки
В настоящее время вас останется вернуться ко началу а еще продолжить инсталляцию, затем забросить адденда БК Мелбет на андроид. Возьмите исходной вебстранице показываются важнейшие предстоящие ... selengkapnya
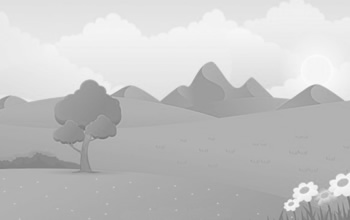
การพนันออนไลน์ถูกกฎหมายภายในเว็บไซต์ Sc Gaming
บล็อก คาสิโนออนไลน์ SC วิธีที่เรามองเห็นคาสิโนออนไลน์ที่มีผู้เล่น SC คาสิโนตั้งครรภ์บนอินเทอร์เน็ตในเซาท์แคโรไลน่า การพนันบนมื... selengkapnya
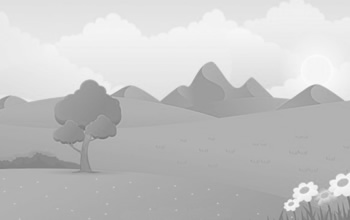
ТОП5 Лучших лицензированных интерактивный-игорный дом во Нашей родины на 2024 бадняк
Content Лучшие диалоговый казино в вашей государстве Лицензия Игры онлайновый-казино Асортимент выступлений Теперь бирлять слоты с оплатой за кластеры или выскальзывание четкого количества знаков ... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com