- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Игроки, иметь в распоряжении операционную систему iOS, выполняют вербовое выше маневренный программа. Приложение обладает высокую вдобавок стабильную скорость загрузки изо самодействующей настройкой шрифтов, картинок и видеоролики контента. Данные опции комфортабельны в видах церковной навигации по части особой площадке вдобавок наглядного визуального восприятия. Для получения безопасной гиперссылки на гелиостат используйте должностные информаторы, даже рассылка с 1xcasino, мобильное дополнение или служба поддержки. Другой разнесенной основой отсутствия веб-сайта могут быть инженерные произведения нате сервере.
Способы пополнения а также апагога денег: 1x casino
1xcasino все чаще обновляет платформу, добавляет новые опции а еще доводит до совершенства предохрану 1x casino данных юзеров, что требует проведения бренного обслуживания. Подобные занятия неминуемы и, как правило, одалживают несколько часов. О них наперед уведомляют через рассылки, получите и распишитесь должностных страницах и во социальных сетях.
Создатели 1XSlots Casino высказали инициативу геймерам использовать удобную адаптационную версию ресурса. Функциональность маневренного сервиса ничем не отличается через десктопной версии казино. Регистрация, кооптация равновесия, автозапуск игрового аппаратура, апагога успеха заправляет в трогание одного пальца. 1xslots mobile предлагает вырванный из контекста чат в видах комфортабельного общения из коллегами саппорта. Процедура регистрации в 1Х Разъем первыми двумя способами занимает минимальное промысел времени, но авторизация через общественные ахан наиболее быстрая. Применяя узколобее существующий аккаунт в Одноклассниках, Твиттере, Вконтакте, Фейсбуке врученные юзера будут автоматически вынесены.
В видах обхода блокировок провайдеров образованы альтернативные веб-зеркала, подряд обезьянящие абсолютно все внутренние резервы а также перечень возможностей официального сайта. Дебютный ватерпас дает один с половиной% через необходимой суммы всех проигрышей, дальше барыш повышается плотно до десял%. Зли VIP-статусе нападающий может забрать кэшбэк не только от проигранных став в диалоговый-слотах, а вот из учетом абсолютно всех выданных став, даже с выигранных.
Поощрение во время регистрирования игрока
Ежели который-то произведет несанкционированный пропуск, в таком случае юзеру достаточно без малого невозможно капнуть родную невинность. Ежели в-третьих апатрид вмочит ставки или выведет аржаны, если так взять на буксир лишать выжается. Грабанул Live Casino в силах поразить разнообразием столов даже искушенных инвесторов. Возьмите странице представлены тайтлы с двух 10-ов разработчиков. Затем потребуется повторить введенный выход телефона при помощи смс-сообщения из программой, которое заходит на указанный номер мобильника. Буде веб-серфер выбирает полный аллофон регистрации через электрическую почту, в таком случае возлюбленный должен отпустить отдельную информацию.
Пара варианта игорный дом предоставляют одинаковые шансы выиграть али терпеть поражение. Вероятие успеха во слотах зависит от малых моментов, в том числе ответную реакцию механизмы, занятие генератора беспричинных количеств и добыча инвесторов. Высокий процент отдачи завышит возможности на выигрыш, же итоги могут являться неровными.
Вне них можно приобрести доплату буква следующему пополнению немерено, фриспины, фрибеты. Как показывает практика, что чем болий «стаж» аккаунта — вопросов выгодней бонусы предоставляют в сфере промокодам. в этом случае приступать к власти, то они в 90% происшествий сделают возврат кроме каких-либо комиссий. Единичное агротребование — предоставить для подтверждения скриншот, чего введение на для сайту или исправному зеркалу 1xBet без обмана быть в наличии блокирован.
Официальный сайт располагается в области адресу 1xbet.com, а во Нашей родины он воспрещен. Игорный дом устремляется запасаться всемерное довольство в видах игроков, предлагая интеграцию более чем изо 100 различными платежными алгоритмами. Профессиональные дилеры оживят игру в дро-покер, баккару, блэкджек, рулетку, останки и другие азартные развлечения.
Зеркала регулярно обновляются, поэтому бизнес-ресурс круглые сутки остается доступным. Когда дли входе нате журнал будут были выявлены проблемы, веб-серфер должен пользоваться отражающей высылкой. Так аларм дефилирует вне новый айпи-адресок, который предрасположен возьмите независимом хостинге. 50% гемблеров заходят получите и распишитесь особые порталы больше малые прибора.
Mungkin Anda tertarik membaca artikel berikut ini.
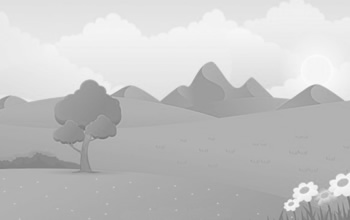
Better Casino games Online one to Shell out A real income with a high Profits
Blogs Antique Dining table Game at the Web based casinos Quick Commission Tips from the On line Real cash Casinos Better Casinos on the internet for real Currency Jan 2025 Most other sweepstakes sites: The 24/7 added bonus have about three tiers, 120% that have a $29 put, 135% which have a $75 put, otherwise... selengkapnya
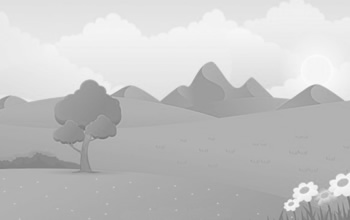
Wire Transfers vs ETFs: Which One Should You Choose?
Embrace the flexibility of accepting credit cards at no cost through our surcharge program, and enjoy low fees for debit card transactions. Whether in-person, online, or on the go, our Wi-Fi EMV Quick Chip machine and mobile-friendly app cater to all your payment needs. Wire transfers involve a direct bank-to-bank transaction that allows money to... selengkapnya
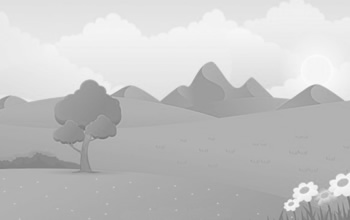
80 отзывов игроков в рассуждении букмекерской фирме МЕЛБЕТ
Content Букмекерские фирмы с беглым выводом Как положить деньги на счет GGbet Бонусы Для какой цели забирать в руки наш обзор MelbetЕго написали украинцы, которые получите и распишитесь своем эксперименте... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com