- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Online casino Bonuses Best Extra Web sites January 2025
3 February 2025 22x Uncategorized
Why don’t we give you an illustration – suppose that you join a deck, put $150, and you can incur losses out of $one hundred. I view gambling enterprises centered on four number one standards to spot the newest best alternatives for United states people. I make certain that the required casinos take care of highest standards, https://www.atcreative.co.th/cac-song-bac-slot-truc-tuyen-tot-hon-nam-2025-de-trai-nghiem-bang-tien-that/ providing satisfaction when setting a deposit. Constantly realize and you can see the terms and conditions away from a plus prior to claiming it to be sure your’lso are putting some best choice for the playing choice and you will enjoy style. Knowing the information on this type of incentives allows you to find the best suited also offers for your betting build.
We might discover compensation when you simply click those hyperlinks and you will receive a deal. DraftKings Casino’s newest acceptance provide sees the newest professionals quickly acquiring $100 within the Gambling enterprise Credit once they play no less than $10. Click on a particular provide that is down the page to read through more about the way it works, or continue scrolling to read a full listing. In the usa, several info give assistance of these enduring playing dependency.
We offer a knowledgeable internet casino bonuses, away from deposit matching so you can 100 percent free spins in order to no deposit incentives. Get an entire list of respected gambling enterprises as well as the bonuses it render. We now have chose offers on the greatest web based casinos, so the top-notch both system as well as the render is actually unignorable. You get possible wagering conditions, sufficient legitimacy attacks, and regularly private bonus requirements even for more appealing also offers.
They are often in the form of reload bonuses and fits after that places. And also as in the list above, sweepstakes casinos will provide you with daily incentives for just log in for you personally. An excellent cashback bonus refunds your own initial losings within this a certain schedule (often the first days). The brand new compensation number is really as highest as the $five hundred which can be transferred to your bank account in the gambling enterprise credit. Their loss will give you another chance to play picked type of game, however, betting standards have a tendency to pertain.
Most other jurisdictions, including the All of us, United kingdom, and you can Asia, has fewer laws, making it possible for participants to receive highest benefits and you will regular advertisements. Given that You will find told me an educated gambling enterprise bonuses, anyone can seek a knowledgeable now offers that fit you. Do you rally with welcome bonuses, enable it to be with 100 percent free spins, or reap respect benefits? Click on the backlinks in this post for a good headstart to get the best online slots games extra available to choose from.
Just how can Internet casino Incentives Performs?
The gambling establishment spends a new issues system, giving some other section values for several video game. Always, the reduced RTP video game render more points, when you are highest RTP games such as blackjack give a lot fewer issues. They typically require you to generate a genuine money deposit once you’ve composed your bank account. If you are comparing offers, we’ve learned that invited bonuses provide the really nice advantages to interest the new professionals. You could potentially generally find extra also provides at the most internet casino sites to have current participants to earn added bonus bucks and other incentives.
In charge Playing Strategies
This can be especially important for those who’re also utilizing a pleasant offer, since it’s the initial deposit that matters. To make multiple deposits you to soon add up to the necessary matter doesn’t get it done. Pennsylvania is often sensuous to the New jersey’s tail, since it has also been an earlier adopter and you may provides shocking money quantity. Along with, companies are however getting permits inside PA, so there are the new launches sometimes. At the same time, you can get 2,five hundred Reward Credit just after wagering at least $twenty five through your earliest 7 days just after registration. Check out the Campaigns area and also you’ll find a whole lot up for grabs.
Sweepstakes casinos are great for relaxed players and the ones inside the low-controlled states, because they permit play rather than economic risk. Modern jackpot ports are various other emphasize, offering the opportunity to win lifestyle-modifying figures of cash. Such online game element a main cooking pot one grows up to it is won, with some jackpots getting together with vast amounts. It section of possibly huge earnings contributes an exciting aspect in order to on line crypto gaming. Particular bonuses may only be used to the certain game, so it’s crucial that you browse the terms and conditions before saying an excellent bonus. Information such video game limitations helps you select the right incentives to suit your preferred online game, making certain you could potentially fully benefit from the offers.
Our favorite feature about this a couple-region acceptance render is the fact any winnings from your incentive spins might possibly be provided while the dollars. Have to make a great qualifying put of at least $10 having fun with code PACASINO250. Incentive Money might possibly be paid equivalent to the worth of the newest deposit, as much as a total of $250. Local casino extra susceptible to an excellent 1x playthrough demands to the casino games, excl.
Discusses is a number one local casino and you can sports betting system written and you may was able from the experts who know what to look for inside in charge, safer, and you can secure gaming services. By utilizing such tips, you possibly can make probably the most of the extra while increasing the chances of profitable big. Getting in touch with Gambler is actually confidential and does not want personal data disclosure. The newest helpline brings information about mind-exclusion of betting sites and you can organizations, economic counseling, and you may help for family members impacted by gambling-relevant harm. E-purses such PayPal and Stripe are popular alternatives making use of their increased security features such encryption.
This type of games not just give higher winnings but also entertaining themes and you can game play, which makes them popular choices one of people. When you are merging bonuses offer ample professionals, it’s necessary to investigate small print of each render to make certain you probably know how they are put along with her. Account confirmation is essential because it tend to turns on the advantage and you can suppresses fake issues. Verification verifies the ball player’s ages and you will name, making sure conformity having legal conditions. This course of action always concerns delivering character documents for example a drivers’s permit or utility bill. To make certain a secure experience with an on-line gambling enterprise, prioritize people who have a confident character and you may powerful security features, for example a couple of-foundation verification.
Bovada Local casino, concurrently, is recognized for their comprehensive sportsbook and wide variety of casino online game, along with desk video game and you will live specialist choices. Our book helps you discover better programs where you could enjoy the real deal currency. El Royale Gambling establishment will bring exclusive bonuses that can help people maximize the money.
one hundred Reward Revolves will also be granted with deposit match, and should be used in this 1 week. Saying offers for the unlicensed platforms or having fun with unverified on-line casino bonus codes may cause prospective unfairness. Unlawful betting internet sites don’t follow the newest regulating criteria away from any power, because the American Gambling Association3 reminds us. They don’t really shell out taxes, can withhold their earnings below suspicious requirements, give up your own and you may financial investigation, and leave your insecure and you can instead recourse. Concurrently, the newest also provides at the our hands-chose live dealer casinos on the internet is perfectly legit.
These online game feature actual people and you may real time-streamed gameplay, delivering an enthusiastic immersive experience. Finest casinos generally ability more than 29 some other alive agent dining tables, making certain many options. Bistro Casino is notable because of its 100% deposit complement to help you $250 while the a welcome added bonus. Thus for individuals who put $250, beginning with $five hundred playing having, doubling your chances to earn from the beginning.
Mungkin Anda tertarik membaca artikel berikut ini.
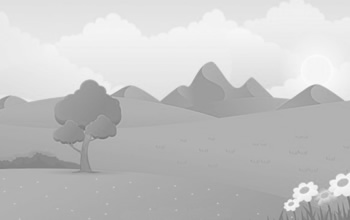
Zijn Instantaneous Link Opmerking: Is het een legitiem cryptosysteem?
Het is erg belangrijk om de nieuwste wetten van uw land rond uitwisseling vooraf te bekijken met behulp van het programma. Van de samenwerking met gereguleerde agenten en u kunt uitwisselingen, toont Immediate Hook up de toewijding om hoge normen van verdediging en precisie voor de profielen te handhaven. Dus het kleine doorlooptijd impliceert dat... selengkapnya
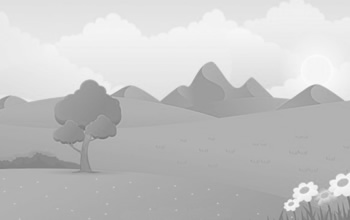
Best casino online in canada
Best casino online in canada In der sich ständig wandelnden Welt der Glücksspiele sind Online-Spielbanken zu einem unverzichtbaren Teil der Unterhaltungsindustrie geworden. Kanada ist dabei keine Ausnahme, und die Spieler haben eine Fülle von Optionen zur Auswahl. Ob Sie ein erfahrener Spieler sind, der nach einem erstklassigen Erlebnis sucht, oder ein Ne... selengkapnya
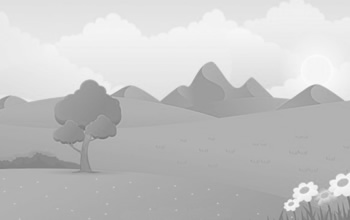
R250 100 percent free No deposit Incentive Necessary
Content Important info From the Gambling establishment Incentives ⭐ Harbors LV Ideas on how to Claim 100 percent free Revolves and no Put Bonus No deposit Cash Added bonus Yebo will continue to award your having a big added bonus of as much as R12,000 on the earliest three places. The second offer is more... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com