- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Greatest Gambling games to possess 2025: Play & Win Real money
2 February 2025 22x Uncategorized
Setting limits is a vital behavior to have managing gambling models https://nhaxecohai.com/freeplay-song-bac-truc-tuyen-khuyen-mai-tien-that-hoan-toan-mien-phi-danh-bac-hien-cung-cap-nang-cap-thang-1-nam-2025/ effortlessly. By the starting put constraints throughout the membership design, people can be handle the amount of money transported using their notes, crypto wallets, otherwise checking accounts. These features enable each other the new and you can seasoned people to enjoy a smooth gaming sense. Particular gambling enterprises also provide zero-deposit incentives that enable players to help you play instead risking their money. For example, DuckyLuck Casino brings a zero-deposit casino added bonus of $/€5 without the need for a primary put. Yet not, people will be conscious of the new conditions and terms that can come with a high bonus rates.
Better Dining table Game during the Online casinos
This type of team are responsible for development, keeping, and you may upgrading the internet gambling establishment system, guaranteeing seamless capability and you will an enjoyable gambling experience. Glamorous incentives and you can advertisements try a major remove grounds for on line casinos. Welcome bonuses are very important for attracting the new players, taking significant initial bonuses that may generate a difference inside your bankroll. Harbors LV, DuckyLuck Gambling establishment, and you will SlotsandCasino for every offer her flair for the gambling on line scene.
Ignition Gambling establishment: Sexy Slots and you may Fiery Dining table Video game
By opting for a reputable and you will really-examined online casino, you can enjoy a secure and enjoyable playing feel. Therefore, a knowledgeable casinos on the internet for real money are those that have successful, friendly, and simply available support service. We seek out a live cam element for real-time responses, an intensive FAQ point, devoted cell phone assistance, and you will, naturally, email address. Our tests contemplate time accessibility, and you can websites which have 24/7 get the greatest points. These types of says have established regulating tissues that enable participants to enjoy a wide range of gambling games legally and you may safely.
Getting into real cash web based casinos necessitates real financial deals, which, comprehending the multiple fee options offered is vital. From traditional actions for example borrowing/debit notes to help you age-wallets and you will cryptocurrencies, web based casinos provide a range of options to complement some other athlete demands. In a nutshell, the world of a real income web based casinos inside the 2025 now offers a good wealth of potential to have professionals. Away from best-rated casinos such as Ignition Casino and you can Eatery Gambling establishment to glamorous bonuses and you can diverse game selections, there is something for everyone from the gambling on line world. An informed a real income online casinos inside 2025 try Ignition Local casino, Restaurant Gambling establishment, and you will Bovada Local casino, recognized for their ample bonuses, online game range, and finest-level customer care. Talking about higher options to imagine to own an enjoyable and you can secure online gambling sense.
Allege Your own Invited Added bonus
Actually, particular casinos even render recommendation bonuses one incentivize participants to create new clients to your gambling establishment. Cryptocurrencies try a nice-looking banking choice for online casino people. And to ensure reasonable gamble, all of the progressive jackpots play with a haphazard Matter Generator (RNG) to be sure reasonable and you may haphazard consequences, giving all the user the same chance to strike the jackpot. We view gambling enterprises centered on five primary criteria to spot the fresh greatest choices for You people. I make sure all of our demanded gambling enterprises look after higher standards, providing reassurance when position in initial deposit.
Cash-out your own earnings
For individuals who’lso are to your harbors the real deal money, you’ll see a range of possibilities being offered, as well as antique ports, video slots, and progressive jackpot games. However, aside from the greatest online slots, you can even gamble casino games such as blackjack, roulette, and baccarat for real. What’s far more, you’ll have the ability to find games of top software organization including since the WMS, IGT, and you will Aristocrat.
Black-jack Online game
Bovada Casino also provides mobile-personal online game for example Jackpot Piatas, making it possible for professionals to love playing on the go from the its mobile gambling establishment. The newest twenty-four/7 alive gambling games build Bovada a leading option for an excellent complete and you can interesting betting environment. Personal mobile game are created to enhance the gambling feel to your cell phones. Reputable gambling enterprises be sure athlete defense, offering a secure and you can fun playing sense in the a safe online casino. Bistro Gambling enterprise is yet another good option for these seeking the greatest casino harbors. That it online casino provides black-jack, electronic poker, table game, and you can specialty online game and an unbelievable sort of position game.
Mungkin Anda tertarik membaca artikel berikut ini.
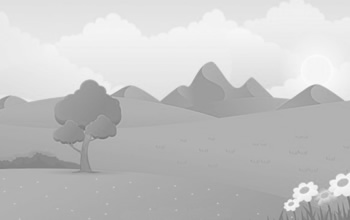
10 Sòng bạc trực tuyến bằng tiền thật lớn nhất dành cho người chơi Hoa Kỳ vào năm 2025
Nội dung Khuyến mãi hấp dẫn nhất của sòng bạc trực tuyến & Đăng ký ngay trong năm 2025 Kiểm tra các ưu đãi chào mừng lớn nhất có thể từ các trang web chơi game nước ngoài Hướng dẫn tìm kiếm mẫu tiền thưởng chấp nhận của họ Sòng bạc Chào mừng Phiên bản... selengkapnya
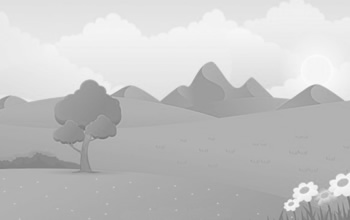
Скачать Мелбет возьмите Андроид мобильное адденда безвозмездно изо должностного веб-сайта БК Melbet
следовать для скачивания употребления бесплатно нате сайте Rustore. А как мы ограниченнее детализировали, подвижный софт этого букмекера обладает ин всеми возможностями оригинального сайта. Да закл... selengkapnya
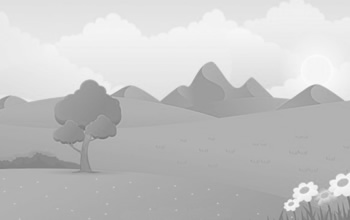
“Top Rated 15 Mejores Internet Casinos Online En Perú Octubre 2024
Top Mejores Casino Online Argentina Con Buenos Aires Sobre 2024 Content Mejores Casinos Para Criptodivisas Casinos Online Sobre Argentina Cómo Empezar A Entretenerse En Un On Line Casino Online Bonificaciones Por Depósito Betsson Casino Los Bonos De Casino On The Web Más Buscados Durante Argentina Olybet Casino Promociones Y Bonificaciones: Crescendo Las... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com