- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Jackpot Area Ontario, Canada: Formal On-line casino Gaming
1 February 2025 24x Uncategorized
Friendly and you can reputable assistance offered twenty four/7 is important to own online casinos. Responsive customer care is extremely important; discover gambling enterprises providing multiple get in touch with steps, along with live chat, cellular phone, and you can email address. Active customer care should include numerous channels such as live talk, email, and you will cell phone choices. Needed mobile casinos inside Canada to possess 2025 offer a massive options out of online game and they are totally enhanced to own cellular have fun with. Bodog stands out because the a top cellular local casino, known for the large-efficiency game geared to mobile pages. If or not because of loyal mobile applications or web browser-based networks, mobile gambling enterprises give a smooth gambling experience.
Expertise Casino Game Types
Cashback offers help the complete gaming feel by providing professionals http://albeliz.com/2025/02/%e0%b8%84%e0%b8%b2%e0%b8%aa%e0%b8%b4%e0%b9%82%e0%b8%99%e0%b8%ad%e0%b8%ad%e0%b8%99%e0%b9%84%e0%b8%a5%e0%b8%99%e0%b9%8c%e0%b9%80%e0%b8%87%e0%b8%b4%e0%b8%99%e0%b8%aa%e0%b8%94%e0%b8%88%e0%b8%a3%e0%b8%b4/ that have a portion of their losings right back, helping decrease losses and you can providing people an additional chance to earn. Particular lower put casinos offer enticing welcome bonuses to have deposits as the low while the $1, after that increasing the pro feel. Get the best sites having higher bonuses, fascinating game, and you will greatest-level protection. Such as, Vegasland offers unique Christmas time bonuses on the joyful-themed slots.
Spin the new reels and try for effective combinations to love fascinating advantages. Of numerous harbors in addition to element progressive jackpots, in which the honor pond increases with every spin up to a lucky user strikes the new jackpot. OnlineGambling.ca (OGCA) try a source designed to simply help its users enjoy wagering and you can local casino gambling. The analysis had been correct during the time of composing, and we cannot be held responsible would be to something alter a short while later. There’s no charge for using our site, and you can relax knowing important computer data is actually safe lined up with this Privacy policy.
Preferred Commission Tricks for A real income Online casinos in the Canada
This enables us to assess your website mediocre, plus the casinos on the high RTPs (always more than 97%) are the best-investing of those. An alternative type of on-line casino game titled an excellent ‘crash game’ could have been continuously becoming more popular in recent months. Basically, there is certainly a good jackpot multiplier that may abruptly stop or ‘crash’ after it has reached a specific area. Freeze game involve a combination of chance but still, as they can be difficult however, worthwhile if you can create to cash-out ahead of the crash. Almost every other variations out of freeze video game were ‘JetX’, that is a-game for which you must accurately anticipate if jets traveling overhead usually freeze. It might take some time for the crash game layout to totally translate into effortless game play, however the on the market types are definitely well worth an attempt.
The new Canadian betting laws may vary anywhere between provinces, but online gambling is not unlawful within the Canada. For the a federal top, zero legislation forbids you against to try out online casino games, however, you will find limits on the playing in the establishments not possessed or authorized because of the an excellent provincial government. The newest Canadian Violent Password are revised inside the 1985, making it possible for parlay-design wagers and you will pari-mutuel betting in the provincial lotteries. It was after altered once again inside the 2021 with Statement C-218, so that unmarried-enjoy sports betting.
Gain benefit from the Flowing Wins mechanics, Haphazard Multipliers, and you will Free Spins, guaranteeing an appealing and rewarding feel amidst the new divine form of that it mythical position thrill. Huge Bass Splash is regarded as Reel Go out Playing’s top fishing ports with an average RTP from 95.67% and you can highest volatility. Whilst position can be found at no cost, it is strongly recommended to try out for real money where a massive 5,000x maximum victory awaits your.
Daniel Smyth have seen the internet poker, gambling enterprise, and you may playing globe out of every position. He’d starred web based poker semi-expertly before doing work at the WPT Journal while the an author and you may publisher. From there, the guy transitioned to help you on the web gaming where the guy’s already been producing expert posts for over ten years. All of our group of reliable casinos is optimized to own mobile gaming, and some actually provide mobile apps to own ios and android gizmos. Antique ports have 3 reels, while multi-reel harbors can have 6, 7, or even more reels, boosting your likelihood of successful.
- As well as harbors, DuckyLuck also provides a wide array of online casino games.
- The result is a great Jackpot Area Gambling establishment you to’s customized to the Ontario-dependent people.
- The new judge years for playing inside the Canada varies by the province, usually put from the 18 or 19 years.
- An alternative sort of online casino game called a great ‘crash game’ has been continuously becoming more popular lately.
- Enjoy innovative gambling alternatives, nice incentives, and regulated platforms one be sure a secure and you can exciting betting experience.
European Roulette
- Appealing offers such local casino bonuses without deposit incentives try a must, to make certain you have the best internet casino feel.
- The minimum deposit is $ten, while the minimal withdrawal is actually $ten for everyone procedures besides the head bank transfer, which includes at least $3 hundred.
- This type of casinos are extremely popular with finances-aware participants who would like to enjoy gambling games as opposed to and make a life threatening money.
- The new agent is actually totally subscribed, protecting your finances and private suggestions.
- These networks usually offer tempting acceptance packages, rewarding you for your 1st financing and you may past.
It’s married that have globe giants for example Microgaming and Progression Gaming, ensuring greatest-notch quality. Which innovative payment system is developing well in popularity one of Canadian professionals, giving a safe and you may effective way to fund its online gambling issues. Looking subscribed and you will controlled web based casinos ensures athlete defense and adherence so you can requirements. Signed up networks focus on user protection and you can reasonable gamble, taking a safe ecosystem to have online gambling. The first step within the determining the safety from web based casinos try to conduct comprehensive background and you may defense monitors.
Quebec’s playing regulations are generally permissive, making it possible for residents to participate many playing items, both in-individual and online. Loto-Québec and operates a unique on the internet gambling system, Espacejeux, which offers many video game as well as casino poker, sports betting, and you will gambling games. If you are private online gambling websites is actually commercially illegal, enforcement against individual people is rare, and some Quebec owners access overseas betting websites. The new province in addition to metropolitan areas an effective emphasis on in charge betting, taking information and help for many who could possibly get produce playing-relevant difficulties. Such online gambling programs make it professionals in order to deposit, choice, and you can winnings a real income thanks to some online game, in addition to online slots, live casino games, and you may progressive jackpots. Bonuses and you will advertisements is a significant draw to have players during the on the internet gambling enterprises, taking extra value and you may improving the gaming feel.
Vintage Black-jack having Commendable Expensive diamonds™
It charm making use of their safer solutions, desirable respect applications, diverse video game choices, and you will sterling customer service characteristics. By the targeting openness and you may affiliate feedback, it continue building the reputation and you may sincerity in the eyes from their people. This type of gambling enterprises do well within the mobile being compatible and you will twenty-four/7 customer care via live chat and you may email, pivotal inside keeping a premier Trustpilot position.
Bodog could have been a reliable label from the gambling on line globe for more than two decades. So it kind of slot online game, as well as popular online slots games, implies that professionals features a varied and fascinating gambling feel. Ensuring secure and safe purchases is the key when it comes to online gambling.
Our analysis means that the brand new playing sites i encourage uphold the new large conditions to own a secure and fun betting feel. Bet365 comes out on top of record as the finest online slot website for successful a real income. It around the world accepted site provides a gigantic set of online game, a person-friendly site, and some of your own fairest wagering requirements in the business. Options are much more limited however the small possibilities features big online game libraries and you will associate-amicable networks playing on the.
Not merely is Jackpot Urban area totally registered, all of our video game because of the leading business, including Video game Worldwide, Pragmatic Enjoy and OnAir™ Activity, is actually authoritative for reasonable gameplay. During the Jackpot Urban area on-line casino we strive to save your safer and safe all the time. Mention a thorough number of internet casino headings on the the mobile program, presenting all of the-time favourites and you can trending titles.
But not, Canadian casinos provide Arbitrary Amount Creator (RNG) casino poker, simulated poker game in which you play alone. If you need alive action, you will additionally see live agent web based poker and therefore simulates the feel of a stone-and-mortar gambling establishment. Just like You casinos on the internet, Canadian betting platforms feature the majority of form of gambling games, with getting more prevalent than the others. We provide a standard listing of game and you may betting choices to cater to one another the fresh and you may educated professionals. Away from slots to web based poker, our very own options assures there’s something you like.
Mungkin Anda tertarik membaca artikel berikut ini.
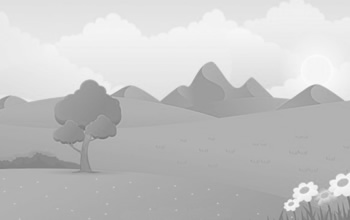
ModelSearcher: The search engine to own OnlyFans
Posts The newest OnlyFans Excursion: Navigating the industry of Exclusive Content writing: @rubypeachxo OnlyFans’ built-searching is first and just works together accurate usernames. ModelSearcher: The major search engines to own OnlyFans Investigating OnlyFans Lookup Potential They’ll be brought in order to an ID verification process that alon... selengkapnya
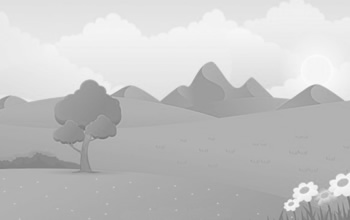
Top Twelve Biggest Casinos In America Size By Square Feet
“Top Ten Largest Casinos In The Usa December 2024 Content Winstar:” “thackerville, Oklahoma (600, 000 Square Feet) Aria Resort & Gambling Establishment In Las Las Vegas, Nevada: 62 01% Five-star Reviews Rome Game Playing Plaza Surprising Facts You Didn’t Know Concerning Gambling Seminole Challenging Rock Hotel And Even Casino In Show... selengkapnya
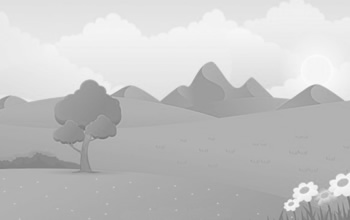
Casino utan svensk person tillstånd 10 Bästa casino inte med Spelpaus
Ni inneha genomgående mindre bra konsumentskydd vilket mest påverkar dej som äge eventuella problem med spelmissbruk. 🎟 Bingo & casino utan svensk licens lotterier – Mindre populära spel, skad finns generellt hos majoriteten från all utländska nätcasinon. ✅ Bristande erfarenhet – Somlig lirar ingen anin att dom lira villig någo utländsk... selengkapnya