- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
A knowledgeable Online slots & Local casino Sense
22 January 2025 22x Uncategorized
Blogs
Choosing an internet casino, you need to be certain of their character and authority among other programs. Better, Enjoy Luck’s fundamental union is to end up being a bar that can act to the strictest criteria. Luck Coins will not processes honours through to the redemption consult has started looked and affirmed. Usually, because of the entire process, you are going to waiting 3 to 5 working days to possess a funds honor redemption from the Chance Coins. As well, you can get an individual membership movie director to help which have questions or support you might need.
- Whether it’s to try out with her in the same room or perhaps from the yourself, you will find some thing for everybody.
- Better, Gamble Fortune’s head union is to become a club that will behave to your strictest criteria.
- Along with, they’ve additional icons to exhibit per game’s volatility, that is a rather nice touch.
- Yes, you’ll find zero-deposit incentive offers to own internet casino ports.
- Unlike antique online casinos, Luck Coins allows professionals lay wagers playing with a couple of digital currencies (Gold coins and you will Fortune Coins), instead of real cash.
Find the extremely funny slot online game right here in the Fortune Games
It’s secure to state that I really liked my personal go out at the Chance Coins Gambling establishment. Even when my personal main objective were to review they, We still got an enjoyable experience dealing with discuss the video game and incentives given. The working platform has highest-high quality harbors, jackpots, seafood game, and you may desk games (while the diversity we have found brief).
Full Writeup on Luck Gold coins Local casino
Here, everyone has a popular classic harbors, along with brand name-the newest launches. All it takes is just one mouse click to explore our very own detailed range and acquire just the right video game for the entertainment demands. Our very own virtual local casino also provides loads of slot machines, all of the with their individual special theme featuring. Be it to play with her in the same room or just by the on your own, we have one thing for all. With more than 1,100 distinct position online game only at Fortune Games, your ideal matches is just just about to happen.
Thankfully, they’ve made it simpler to allege a full incentive instead of wishing weeks. Allow me to determine the way to earn much more money to help you continue to play. While you are wanting to is actually simply in charge betting, the new Chance gambling enterprise try a location that’s sure in order to meet the means.
Of numerous well-known banking choices are mastercard dumps, eWallets, cryptocurrencies, and you can prepaid cards used to pay for your account when to experience online casino harbors. On-line casino harbors provide participants a quick-paced, fun playing sense. They could even be appreciated at home otherwise on the run through mobile phones. Online position betting is actually underneath the attentive vision from authorities, and you will internet sites encrypt your computer data for optimum security.
Furthermore, getting profiles having updated analysis and you can books from most recent gaming news often enable him or her to make smart choices while playing online slots. Prior to looking an on-line gambling enterprise, ensure that you conduct your own due diligence. Though there are many dependable betting websites giving real money slot online game to possess British players, only wild.io casino some of them will be trusted. You need to ensure the local casino is registered and you can managed because of the a reputable governing body and contains a good reputation to possess delivering reasonable and sincere game play. You can find all kinds of games harbors online that you can pick from within the uk. If you are looking on the greatest internet casino experience in an excellent big set of video game, look no further than Fortune Game.
The video game have been designed getting compatible with your entire favorite gizmos, along with android and ios mobile phones/tablets; Screen Personal computers; Macs; and you will Linux machines. You can enjoy all of our video game thanks to an internet browser otherwise by downloading an application – the possibility is actually your. The newest enthralling action out of Ruby Chance’s online casino games isn’t limited by your pc. In case your preference is always to twist reels or wager during the tables, our mobile video game are common you desire.
To the capacity for internet casino harbors, you might use your time and effort without the restrictions.
The site tons easily, no matter where you are in the usa, and you will navigating it’s easy. Everything required is on the new website, away from financial choices to incentives and you will likely to the overall game list. The fresh sweepstakes local casino also features an FAQ web page covering the really preferred inquiries.
You might discuss certain online game and find out those interest really for you. Up coming, after you getting confident in the alternatives(s), carry it up a level by the playing with real money and you will maybe even hit some jackpots. Are you wanting to discover what can make Fortune Video game stand out of the remaining portion of the online casino industry?
It virtual globe will end up more enjoyable and you can dazzling with this biggest harbors that may strike possibly the really fanciful client. Visit our very own magnificent club chance gambling enterprise, and this captivates your focus from the basic second. Because the Luck Coins Gambling enterprise try a play-for-fun system, it will not have antique certification. That said, the video game provided provides RNGs (Random Count Machines) followed, ensuring haphazard efficiency and equity. A casino’s acceptance added bonus ‘s the earliest bonus people put their eyes on, so this is in which I began my personal Chance Coins journey. When the there’s a game you to’s already supposed widespread, you’re bound to view it on this site.
And observe that before you make the first redemption, you ought to make certain your account because of the submission an authorities-given ID, proof of address, and you will a financial statement. You can stimulate this type of just in case necessary to stop a lot of playtime training. Even though you’re convinced regarding the possibility, keep in mind that there is always a component of chance during the play. The impeccable image and you may voice construction create a good lifelike surroundings one transfers you to Las vegas. Your details is definitely steadfastly protected with your safe encoding, which means that your information that is personal stays safe.
It is important that you make sure to run comprehensive search when shopping for a dependable gambling establishment playing slot video game. A quick Browse can give you strategies, but definitely comprehend consumer reviews prior to buying one program. Having Luck Video game, our very own characteristics are highly rated, and we offer a memorable gaming feel – making us stand out from almost every other gambling enterprises. If the advanced support service and you will electrifying gameplay number extremely for you, then there’s zero better option than Luck Video game.
Launched inside the 2022, it gambling establishment performs lower than an excellent sweepstakes design which can be a good replacement genuine-money betting locations. In charge playing practices is actually encouraged at the on-line casino slots other sites, this is why they will normally have founded-within the automatic restriction or notice-ban have. Not missing out on an excellent game due to day commitments or inclement weather. So long as need to be concerned regarding the getting the right outfit or searching for parking when travelling well away from home while the everything is available at hand. Enjoy full comfort with this on the web slot games each time, everywhere.
Mungkin Anda tertarik membaca artikel berikut ini.
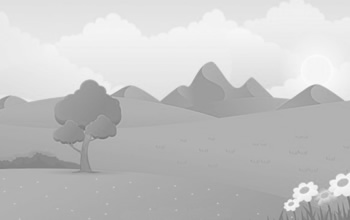
คาสิโนออนไลน์รายได้จริงที่ดีกว่าในแคนาดา มกราคม 2025 CC
คุณสามารถเพลิดเพลินไปกับวิดีโอเกมยอดนิยม เช่น Activities Past Wonderland, Money Miss Real Time และ Quantum Roulette https://2020.pdaf.net/?p=193891 คาสิโนออนไลน์ขนาดใหญ่ของแคนาดานำเสนอควา... selengkapnya
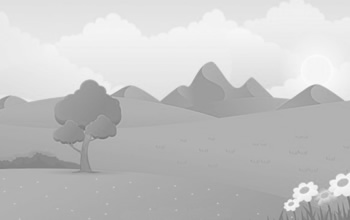
Safari Slots Free online Gambling enterprise Games from the Endorphina
Content Available Commission Choices Free Bonus Bucks A little more about No deposit Bonuses Much more No-deposit Incentives to try Commitment apps tend to element extra pros such private tournaments and you will unique marketing and advertising situations. Such software award you to suit your went on patronage, providing you more possibilities to win and... selengkapnya
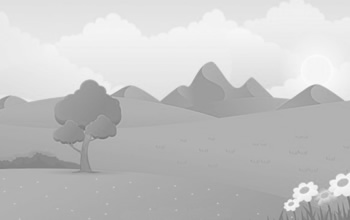
Zakłady Bukmacherskie ️ Obstawiaj Zakłady Online Unces Vulkanbet
Betcris Zakłady Bukmacherskie Legalny Bukmacher Online Content Typuj Mecze W Konkursie Liga Angielska ⚽jakie Dyscypliny Wonderful I Wydarzenia Można Obstawiać W 1xbet? Sprawdź Kursy Bukmacherskie Em Dziś Blog – Artykuły, Typy, Wiadomości Ze Świata Sportu Zakłady Bukmacherskie Betcris – Legalny Bukmacher On The Web” “[newline]ku... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com