- Hubungi Kami via WhatsApp dan Dapatkan DISKON
- Special Promo Di Bulan Ini.
Content
Choosing casinos you to definitely comply with state laws is key to making sure a secure and equitable gaming sense. Selecting the right crypto gambling enterprises in it an intensive review of more fifty websites, making certain a clear and you may enjoyable betting sense to own players. Area of the criteria provided protection, games variety, reasonable gamble, and you will exchange price.
- Today, there are other than several gambling establishment websites doing work legitimately inside the usa, however it is constantly good to look at the country’s position.
- Merely just after we now have starred extensively during the an on-line casino can we make the last opinions and you will ratings.
- Now the main BetMGM network, it’s got an impressive collection more than cuatro,100 online game, in addition to harbors, alive specialist game, desk online game, and a wide range of jackpot ports.
The net gambling landscape in the united states is varied, composed more of state-height laws and regulations instead of unified federal legislation. If you are specific states has totally welcomed the field of casinos on the internet, anyone else features tight constraints up against they. In a number of You says even, there are downright bans to the gambling on line. I become familiar with the safety standards of every casino to ensure you to it bring detailed procedures to protect your data.
Caesars Sportsbook & Gambling enterprise
Professionals can also enjoy various ports, real time gambling games such as blackjack and you may roulette, desk game, and much more. Best on the internet crypto casinos render smaller winnings and lower fees compared to conventional casinos, popular with tech-experienced users. But not, they often times work with an appropriate gray area, contrasting to your dependent regulations from antique online casinos and you can crypto local casino websites, as well as individuals crypto playing web sites. Incentives and you can advertisements try a serious mark to own players in the crypto online casinos.
First-Day Put Incentive
A lot of safe Bitcoin gambling enterprises try on the web, although not folks are equivalent. The newest easiest Bitcoin casinos have good certificates, strong security features, https://maana.app/cac-song-bac-truc-tuyen-bang-tien-that-tot-nhat-cho-nguoi-tham-gia-nam-2025/ legitimate customer service, and reasonable video game. Evaluating most other participants is a superb treatment for verify that a webpages is actually dependable. To possess brand new Bitcoin gambling enterprises that may n’t have reviews yet ,, take some time to complete your research and get careful to protect oneself online.
Slot Games of the Day
Position online game will be the top treasures of internet casino gaming, offering professionals a way to earn large that have modern jackpots and you will stepping into many different layouts and you will game play aspects. Understanding how to play sensibly concerns recognizing the signs of betting dependency and seeking let when needed. Web based casinos provide info for the in charge betting, as well as tips for acknowledging problem gambling and you may alternatives for self-exception.
Today they offer headings in order to regulated gambling establishment workers in the over twenty-five jurisdictions, along with Diamond Vortex, Riddle Reels, and you will Rally 4 Riches. Several award-effective software team are engaged in a continual find it hard to discharge by far the most fun the new online game yearly. People say becoming forewarned is going to be forearmed, and no place performs this ring truer compared to the industry of gambling enterprise incentives. Very, take your time to fully discover what is actually a part of for every provide.
Exactly what are the advantages of choosing cryptocurrency for gambling on line?
With its relaxed design and you may varied collection from video game, Restaurant Gambling enterprise makes for the greatest warm part for online gaming. As well as for web based poker participants, there’s the brand new adventure from large-power poker bed room that have options for anonymous gamble and you will a choice out of competitions. Harbors LV is not far at the rear of, appealing people that have an excellent one hundred% match incentive as much as $dos,100000, and the attract from 20 100 percent free spins.
Other says for example California, Illinois, Indiana, Massachusetts, and New york are expected to pass similar laws in the future.
A real income Gambling games
But not, once doing a bit of lookup I really believe they’s crucial that you notice as the business appears to be powering efficiently today, there are things in the past. Inside 2019, Ladbrokes Coral Class have been needed to shell out a great £5.9m okay for previous failings in the anti-currency laundering and you may public responsibility, with regards to the British Gaming Fee. Professionals have a go away from successful a fortune from the webpages’s MGM Millions ability for the super jackpot often status in the over £20 million. For individuals who mouse click backlinks with other sites in this post, we are going to secure fee. In charge gambling setting residing in control of the betting and you will staying they fun instead of letting it harm your finances or really-being.
There is certainly sufficient assortment to fulfill the brand new choices of all of the types of bettors. After an extensive journey from the realms from online casino betting, it becomes obvious that the industry within the 2025 are surviving that have options for all types of player. On the finest internet sites giving generous greeting packages on the varied assortment of game and you will safer percentage actions, online gambling has never been a lot more accessible otherwise fun. Which part provides together the main issues chatted about from the article and leave members with a last said to promote its upcoming playing endeavors.
Mungkin Anda tertarik membaca artikel berikut ini.
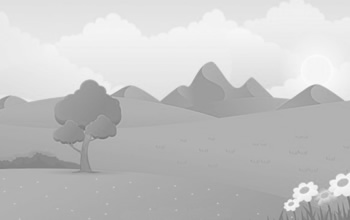
Play Real money Ports & Desk Game
Posts A real income Online slots games from the Bally Bet Gambling enterprise Keno, Bingo, Desk Video game & Video poker Much more Video game Huge Jackpot Slot Game Sure, Bally Gambling enterprise will bring a devoted Bally application for both Android os and you may ios users. The new application is designed to provide... selengkapnya
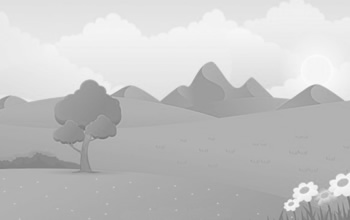
“букмекерская Контора Betboom Ставки На Спорт Онлайн В России!
Ставки На Спорт Букмекерская Контора «балтбет» Content Тенниси На Пожар без Маржи Американцы Возмущены Линиями На переломные В Лос-анджелесе Бонусы И Акции Коэффициенты И Маржа Ставки На Спорт Как в... selengkapnya
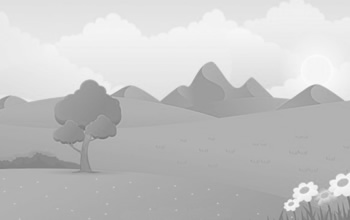
Online Canlı Bahis, Casino Sitesi
Betgit Giriş: Yüksek Oranlar Canlı Bahis Sitesi Gambling Establishment Oyunları” Content Başarı Bet Casino’nun Temel Özellikleri Casino Metropol Müşteri Hizmeti Casinoroyal Nasıl Bir Internet Site? Basaribet’in Benzersiz Oyun Çeşitliliği Casinoroyal Casino Slot Ekstra Bonuslar Jackpot Oyunları Metropol Casino Veya Casino Metropol ... selengkapnya
Kontak Kami
Apabila ada yang ditanyakan, silahkan hubungi kami melalui kontak di bawah ini.
-
Hotline
082325487331 -
Whatsapp
082325487331 -
Instagram
nusapenidalink -
Email
awirbujadewagede@gmail.com